Steps to Create a custom WordPress blog theme with the following features like widgets, theme customizer options, or custom post types
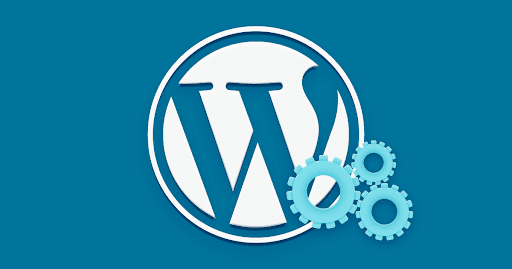
Below is an inβdepth example and explanation of how to create a custom blog WordPress theme that includes key features such as widget areas, Theme Customizer options, and custom post types. The guide is organized into a suggested file/folder structure, code examples in PHP, HTML, JS, and CSS, and explanations for each key point.
1. File and Folder Structure
A well-organized theme might have a structure like this:
myblogtheme/
βββ assets/
β βββ css/
β β βββ custom.css // Additional CSS (optional)
β βββ js/
β βββ script.js // Custom JavaScript
βββ inc/
β βββ customizer.php // Theme Customizer settings
β βββ widgets.php // Widget registration and setup
β βββ custom-post-types.php// Custom post type registration
βββ template-parts/
β βββ content.php // Reusable content template parts
βββ header.php // Contains <head> and top navigation markup
βββ footer.php // Contains footer markup and closing HTML
βββ index.php // Main template file
βββ sidebar.php // Sidebar template, typically used for widgets
βββ functions.php // Enqueues styles/scripts and includes inc files
βββ style.css // Theme information header and main stylesheet
Key Points:
- Root Files:
style.css
holds theme meta-information;functions.php
enqueues assets and includes additional PHP files.- Template Files:
header.php
,footer.php
,index.php
, etc., structure your pages.- Assets: Separate folders for CSS and JS keep your code modular.
- inc Folder: Contains extra functionality such as customizer settings, widgets, and custom post types.
2. Theme Files & Code Examples
a. style.css
This file begins with a header comment block that tells WordPress about your theme.
/*
Theme Name: My Blog Theme
Theme URI: http://example.com/myblogtheme
Author: Your Name
Author URI: http://example.com
Description: A custom blog theme featuring widgets, customizer options, and custom post types.
Version: 1.0
License: GNU General Public License v2 or later
License URI: http://www.gnu.org/licenses/gpl-2.0.html
Text Domain: myblogtheme
*/
Explanation:
This header block is required. WordPress reads it to display theme details in the dashboard.
b. functions.php
This file sets up your theme’s functionality. It registers widget areas, enqueues styles and scripts, and includes additional functionality from the /inc
folder.
<?php
// functions.php
// Enqueue styles and scripts
function myblogtheme_enqueue_scripts() {
wp_enqueue_style('myblogtheme-style', get_stylesheet_uri());
wp_enqueue_style('myblogtheme-custom', get_template_directory_uri() . '/assets/css/custom.css');
wp_enqueue_script('myblogtheme-script', get_template_directory_uri() . '/assets/js/script.js', array('jquery'), '1.0', true);
}
add_action('wp_enqueue_scripts', 'myblogtheme_enqueue_scripts');
// Add theme support for features
function myblogtheme_setup() {
add_theme_support('post-thumbnails'); // Featured images
add_theme_support('custom-logo');
add_theme_support('title-tag');
// Add other supports as needed
}
add_action('after_setup_theme', 'myblogtheme_setup');
// Include additional files for widgets, customizer, and custom post types
require get_template_directory() . '/inc/widgets.php';
require get_template_directory() . '/inc/customizer.php';
require get_template_directory() . '/inc/custom-post-types.php';
?>
Explanation:
- Enqueuing assets: Ensures CSS and JS load correctly.
- Theme support: Enables features such as post thumbnails and title tags.
- Includes: Modularizes your code by splitting widgets, customizer, and custom post type code into separate files.
c. inc/widgets.php
This file registers widget areas (sidebars) so users can add widgets via the WordPress dashboard.
<?php
// inc/widgets.php
function myblogtheme_widgets_init() {
register_sidebar( array(
'name' => __('Sidebar', 'myblogtheme'),
'id' => 'sidebar-1',
'description' => __('Add widgets here to appear in your sidebar.', 'myblogtheme'),
'before_widget' => '<section id="%1$s" class="widget %2$s">',
'after_widget' => '</section>',
'before_title' => '<h2 class="widget-title">',
'after_title' => '</h2>',
) );
}
add_action('widgets_init', 'myblogtheme_widgets_init');
?>
Explanation:
This registers a sidebar where widgets can be added. The markup wrappers (before_widget
, etc.) let you style widgets with CSS.
d. inc/customizer.php
This file adds options to the Theme Customizer, allowing users to change theme settings (e.g., logo, colors).
<?php
// inc/customizer.php
function myblogtheme_customize_register($wp_customize) {
// Add a section for theme options
$wp_customize->add_section('myblogtheme_options', array(
'title' => __('Theme Options', 'myblogtheme'),
'priority' => 30,
));
// Add a setting for the header background color
$wp_customize->add_setting('header_bg_color', array(
'default' => '#ffffff',
'sanitize_callback' => 'sanitize_hex_color',
));
// Add a control to change header background color
$wp_customize->add_control(new WP_Customize_Color_Control($wp_customize, 'header_bg_color_control', array(
'label' => __('Header Background Color', 'myblogtheme'),
'section' => 'myblogtheme_options',
'settings' => 'header_bg_color',
)));
}
add_action('customize_register', 'myblogtheme_customize_register');
?>
Explanation:
- Sections & Settings: You create a section (
myblogtheme_options
) for custom theme settings and add a color setting for the header. - Controls: A color picker is added so the user can choose a header background color.
- Sanitization: Ensures that user inputs (like color codes) are safe.
e. inc/custom-post-types.php
This file registers a custom post type. Although WordPress already provides the “post” type for blogs, you might want a secondary type (for example, “Portfolio” or “Review”).
<?php
// inc/custom-post-types.php
function myblogtheme_register_custom_post_types() {
$labels = array(
'name' => _x('Portfolios', 'post type general name', 'myblogtheme'),
'singular_name' => _x('Portfolio', 'post type singular name', 'myblogtheme'),
'menu_name' => _x('Portfolios', 'admin menu', 'myblogtheme'),
'name_admin_bar' => _x('Portfolio', 'add new on admin bar', 'myblogtheme'),
'add_new' => _x('Add New', 'portfolio', 'myblogtheme'),
'add_new_item' => __('Add New Portfolio', 'myblogtheme'),
'new_item' => __('New Portfolio', 'myblogtheme'),
'edit_item' => __('Edit Portfolio', 'myblogtheme'),
'view_item' => __('View Portfolio', 'myblogtheme'),
'all_items' => __('All Portfolios', 'myblogtheme'),
);
$args = array(
'labels' => $labels,
'public' => true,
'has_archive' => true,
'supports' => array('title', 'editor', 'thumbnail', 'excerpt'),
'rewrite' => array('slug' => 'portfolios'),
'show_in_rest' => true, // For Gutenberg support
);
register_post_type('portfolio', $args);
}
add_action('init', 'myblogtheme_register_custom_post_types');
?>
Explanation:
- Custom Post Type: This code creates a “Portfolio” post type.
- Arguments: Defines labels, supports (such as title, editor, thumbnail), and settings like URL rewrite and REST API support.
f. Template Files
header.php
Contains the opening HTML markup, meta tags, and the navigation menu.
<!DOCTYPE html>
<html <?php language_attributes(); ?>>
<head>
<meta charset="<?php bloginfo( 'charset' ); ?>">
<meta name="viewport" content="width=device-width, initial-scale=1">
<?php wp_head(); ?>
</head>
<body <?php body_class(); ?>>
<header id="masthead" style="background-color: <?php echo get_theme_mod('header_bg_color', '#ffffff'); ?>;">
<div class="site-branding">
<?php if (function_exists('the_custom_logo')) { the_custom_logo(); } ?>
<h1 class="site-title"><a href="<?php echo esc_url(home_url('/')); ?>"><?php bloginfo('name'); ?></a></h1>
</div>
<nav id="site-navigation">
<?php wp_nav_menu(array('theme_location' => 'primary')); ?>
</nav>
</header>
Explanation:
- Dynamic Values: Retrieves settings such as the header background color (set via the customizer) and displays the custom logo.
- Navigation: Uses
wp_nav_menu
to output a menu that can be managed from the dashboard.
index.php
This is the main template file that controls the layout of your homepage or blog index.
<?php get_header(); ?>
<div id="primary" class="content-area">
<main id="main" class="site-main">
<?php
if ( have_posts() ) :
while ( have_posts() ) : the_post();
// Include a template part for the content. You can also use get_template_part('template-parts/content', get_post_format());
?>
<article id="post-<?php the_ID(); ?>" <?php post_class(); ?>>
<header class="entry-header">
<?php the_title('<h2 class="entry-title"><a href="' . esc_url(get_permalink()) . '">', '</a></h2>'); ?>
</header>
<div class="entry-content">
<?php the_excerpt(); ?>
</div>
</article>
<?php
endwhile;
the_posts_navigation();
else :
echo '<p>No posts found</p>';
endif;
?>
</main>
</div>
<?php get_sidebar(); ?>
<?php get_footer(); ?>
Explanation:
- Loop: The standard WordPress loop to display posts.
- Template Tags: Functions like
the_title()
,the_excerpt()
, andthe_permalink()
are used to output post data.
sidebar.php
Displays the widget area registered in inc/widgets.php
.
<aside id="secondary" class="widget-area">
<?php if ( is_active_sidebar( 'sidebar-1' ) ) : ?>
<?php dynamic_sidebar( 'sidebar-1' ); ?>
<?php endif; ?>
</aside>
Explanation:
This code checks if the sidebar has active widgets and then displays them using dynamic_sidebar
.
footer.php
Closes the HTML structure and calls wp_footer()
.
<footer id="colophon" class="site-footer">
<div class="site-info">
<p>© <?php echo date("Y"); ?> <?php bloginfo('name'); ?></p>
</div>
</footer>
<?php wp_footer(); ?>
</body>
</html>
Explanation:
- Dynamic Date: Displays the current year dynamically.
- wp_footer(): This hook is critical for plugins and scripts to be inserted before the closing
</body>
tag.
g. assets/js/script.js
A simple JavaScript file to add any dynamic interactions.
// assets/js/script.js
document.addEventListener('DOMContentLoaded', function() {
console.log("My Blog Theme is ready!");
// Add additional JS functionality here.
});
Explanation:
This file demonstrates how to include custom JavaScript. It logs a message when the DOM is ready.
h. assets/css/custom.css
Additional custom CSS for your theme.
/* assets/css/custom.css */
body {
font-family: Arial, sans-serif;
line-height: 1.6;
background-color: #f9f9f9;
}
.site-title a {
text-decoration: none;
color: #333;
}
/* Widget styling */
.widget {
margin-bottom: 20px;
padding: 10px;
background: #fff;
border: 1px solid #ddd;
}
Explanation:
This file allows you to style specific parts of your theme beyond the basic styles in style.css
.
3. How It All Works Together
-
Theme Initialization:
When your theme is activated, WordPress readsstyle.css
for meta information andfunctions.php
to set up scripts, theme supports, and include additional functionality. -
Customizer Options:
The customizer settings frominc/customizer.php
let users pick options (e.g., header background color), which are then output in the header usingget_theme_mod()
. -
Widget Areas:
The sidebar defined ininc/widgets.php
allows widgets to be added via the admin interface and rendered insidebar.php
. -
Custom Post Types:
By registering a custom post type (Portfolio) ininc/custom-post-types.php
, you extend WordPress functionality so that users can create additional content types separate from regular posts. -
Template Files:
Files likeheader.php
,index.php
, andfooter.php
build the front-end layout, whileassets/
holds your CSS/JS resources.
4. Final Notes
- Modularity: Breaking functionality into separate files (in the
/inc
folder) keeps your theme code organized and maintainable. - Dynamic Content: Use WordPress functions (like
wp_nav_menu()
,the_post()
,get_theme_mod()
) to pull dynamic content from the database. - Extensibility: You can expand this foundation by adding more customizer options, additional widget areas, or more custom post types as needed.
This example provides a solid starting point for creating a custom blog WordPress theme with widgets, Theme Customizer options, and custom post types. You can build upon this structure to meet your specific design and functionality requirements.