How to Optimize JavaScript for Better Web Performance
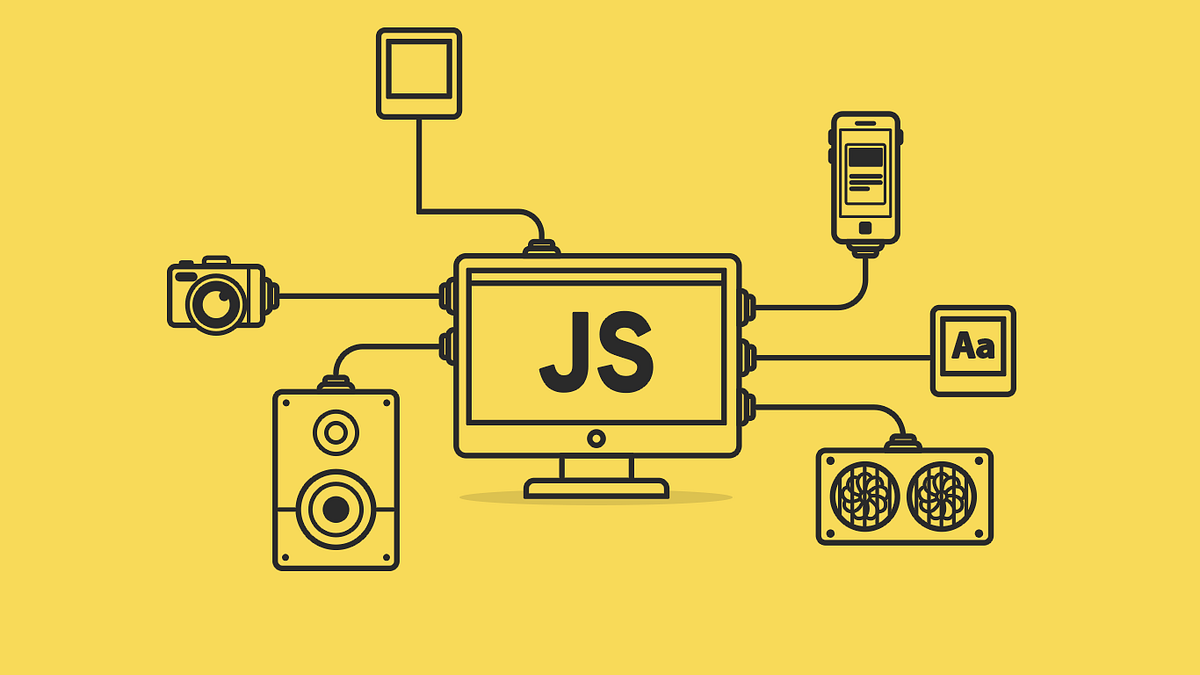
Here's a detailed guide on optimizing JavaScript for better web performance, including explanations, keywords, and examples.
JavaScript plays a crucial role in modern web applications, but excessive or inefficient JavaScript can lead to slow loading times, unresponsive UI, and poor user experience. Optimizing JavaScript helps improve website speed, responsiveness, and SEO ranking.
Below are the best practices for optimizing JavaScript:
1. Minify and Compress JavaScript Files
Keyword: JavaScript minification, file compression, reduce file size
Explanation: Minification removes unnecessary spaces, comments, and redundant code from JavaScript files, reducing their size and improving load speed. Compression further reduces bandwidth usage.
Example:
Using Terser (a popular minification tool):
npx terser script.js -o script.min.js -c -m
Using Gzip Compression in .htaccess
for Apache servers:
<IfModule mod_deflate.c>
AddOutputFilterByType DEFLATE application/javascript
</IfModule>
2. Use Asynchronous and Deferred Loading
Keyword: Async, Defer, non-blocking JavaScript
Explanation: By default, JavaScript blocks HTML parsing. Using async
or defer
ensures the script does not delay rendering.
- Async: Loads the script asynchronously but executes it immediately after loading.
- Defer: Loads the script asynchronously and executes it after HTML parsing.
Example:
<script src="script.js" async></script>
<script src="script.js" defer></script>
Use async
for scripts that don’t depend on others (e.g., analytics), and defer
for scripts that should run after HTML is parsed.
3. Reduce DOM Manipulation
Keyword: Virtual DOM, batch updates, optimize reflows
Explanation: Frequent updates to the DOM cause reflows and repaints, slowing down the page. Optimize by batching DOM updates and using fragment techniques.
Example:
Instead of:
const list = document.getElementById("list");
for (let i = 0; i < 1000; i++) {
const li = document.createElement("li");
li.textContent = `Item ${i}`;
list.appendChild(li);
}
Use DocumentFragment:
const list = document.getElementById("list");
const fragment = document.createDocumentFragment();
for (let i = 0; i < 1000; i++) {
const li = document.createElement("li");
li.textContent = `Item ${i}`;
fragment.appendChild(li);
}
list.appendChild(fragment);
4. Implement Lazy Loading for JavaScript
Keyword: Lazy loading, optimize page speed, load on demand
Explanation: Load JavaScript files only when they are needed to improve initial page load time.
Example: Lazy load a script using IntersectionObserver
:
function loadScript(url) {
let script = document.createElement("script");
script.src = url;
document.body.appendChild(script);
}
const observer = new IntersectionObserver((entries) => {
if (entries[0].isIntersecting) {
loadScript("heavy-script.js");
observer.disconnect();
}
}, { threshold: 1 });
observer.observe(document.getElementById("loadTrigger"));
5. Optimize Loops and Functions
Keyword: Efficient loops, avoid unnecessary calculations
Explanation: Use efficient loops (forEach
, map
, reduce
), avoid redundant calculations, and minimize function calls inside loops.
Example:
Instead of:
for (let i = 0; i < array.length; i++) {
console.log(array[i]);
}
Use:
array.forEach(item => console.log(item));
6. Avoid Memory Leaks
Keyword: Memory management, event listeners, garbage collection
Explanation: Unnecessary variables, retained DOM references, and event listeners can cause memory leaks.
Example: Remove event listeners when no longer needed:
const button = document.getElementById("btn");
function handleClick() {
console.log("Clicked");
}
button.addEventListener("click", handleClick);
// Remove event listener to prevent memory leak
button.removeEventListener("click", handleClick);
7. Use Code Splitting and Bundle Optimization
Keyword: Webpack, dynamic import, split bundle
Explanation: Instead of loading all JavaScript at once, use code splitting to load only necessary parts.
Example: Using dynamic import:
document.getElementById("loadModule").addEventListener("click", async () => {
const module = await import("./module.js");
module.default();
});
8. Use a Content Delivery Network (CDN)
Keyword: CDN, global caching, reduce latency
Explanation: A CDN caches JavaScript files in multiple locations worldwide, reducing load time.
Example: Load jQuery from a CDN instead of a local file:
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
9. Optimize Event Delegation
Keyword: Event delegation, improve performance, fewer event handlers
Explanation: Instead of adding event listeners to multiple elements, attach a single listener to a parent element.
Example:
Instead of:
document.getElementById("button1").addEventListener("click", handleClick);
document.getElementById("button2").addEventListener("click", handleClick);
Use event delegation:
document.getElementById("container").addEventListener("click", (event) => {
if (event.target.matches(".btn")) {
console.log("Button clicked:", event.target);
}
});
10. Reduce Third-Party Dependencies
Keyword: Avoid unnecessary libraries, optimize external scripts
Explanation: Using too many third-party libraries can slow down performance. Replace large libraries with vanilla JavaScript or lightweight alternatives.
Example: Instead of using jQuery for simple tasks:
$("#element").addClass("active");
Use vanilla JavaScript:
document.getElementById("element").classList.add("active");
Optimizing JavaScript is crucial for a fast and efficient web experience. By following these techniques—minifying files, using asynchronous loading, reducing DOM manipulations, and optimizing loops—you can significantly enhance website performance.