How to Create a Flickity Slider in JavaScript with Thumbnail Navigation
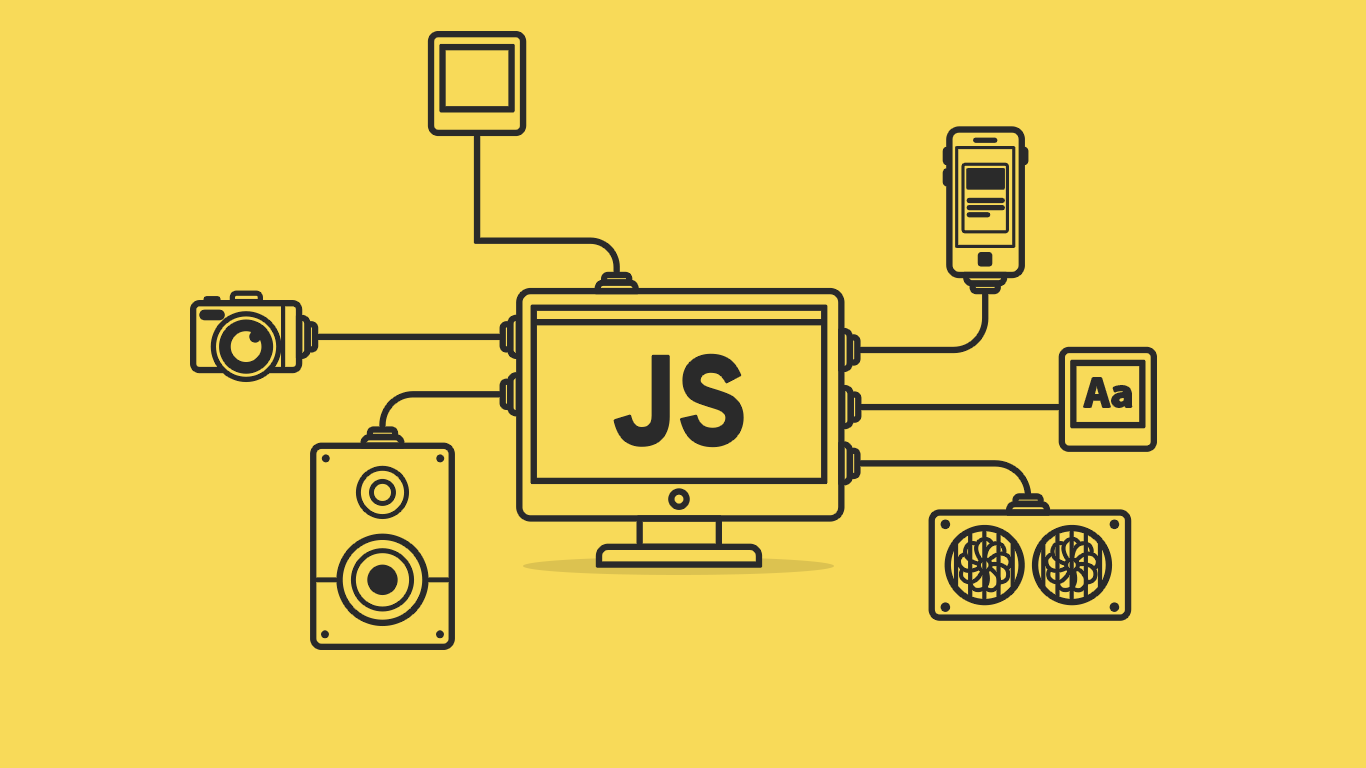
Flickity is a popular JavaScript library for creating responsive and touch-friendly sliders. It supports various features, including thumbnail navigation, which allows users to click on small preview images to navigate the main carousel. Below, I'll walk you through the process of creating a Flickity slider with thumbnail navigation, explaining each key step.
1. Include Flickity Library
Before using Flickity, you need to include its CSS and JavaScript files in your project. You can either use a CDN or install it via npm.
Option 1: Using CDN
Add the following links to your HTML file:
<!-- Flickity CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/flickity/2.3.0/flickity.min.css">
<!-- Flickity JS -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/flickity/2.3.0/flickity.pkgd.min.js"></script>
Option 2: Installing via npm
If you are using a package manager, install Flickity using:
npm install flickity
Then, import it in your JavaScript file:
import Flickity from 'flickity';
import 'flickity/css/flickity.css';
2. Create HTML Structure for the Slider
We need two carousels:
- Main carousel – Displays large images.
- Thumbnail carousel – Small images for navigation.
Here’s the basic HTML structure:
<div class="carousel-main">
<div class="carousel-cell"><img src="image1.jpg" alt="Image 1"></div>
<div class="carousel-cell"><img src="image2.jpg" alt="Image 2"></div>
<div class="carousel-cell"><img src="image3.jpg" alt="Image 3"></div>
<div class="carousel-cell"><img src="image4.jpg" alt="Image 4"></div>
</div>
<div class="carousel-nav">
<div class="carousel-cell"><img src="image1-thumb.jpg" alt="Thumbnail 1"></div>
<div class="carousel-cell"><img src="image2-thumb.jpg" alt="Thumbnail 2"></div>
<div class="carousel-cell"><img src="image3-thumb.jpg" alt="Thumbnail 3"></div>
<div class="carousel-cell"><img src="image4-thumb.jpg" alt="Thumbnail 4"></div>
</div>
3. Style the Slider with CSS
To make the slider look neat, apply some basic CSS:
.carousel-main {
width: 80%;
margin: auto;
}
.carousel-cell img {
width: 100%;
height: auto;
display: block;
}
.carousel-nav {
width: 60%;
margin: 20px auto 0;
display: flex;
justify-content: center;
}
.carousel-nav .carousel-cell {
width: 60px;
margin: 0 5px;
cursor: pointer;
opacity: 0.6;
}
.carousel-nav .carousel-cell.is-selected {
opacity: 1;
border: 2px solid #000;
}
4. Initialize Flickity in JavaScript
Now, we need to initialize both the main carousel and the thumbnail navigation.
document.addEventListener("DOMContentLoaded", function () {
var mainCarousel = new Flickity(".carousel-main", {
cellAlign: "center",
contain: true,
wrapAround: true,
pageDots: false
});
var navCarousel = new Flickity(".carousel-nav", {
asNavFor: ".carousel-main",
contain: true,
pageDots: false,
prevNextButtons: false,
cellAlign: "center"
});
});
5. Breakdown of Key Points
1️⃣ Setting up Flickity
- We include Flickity's CSS and JavaScript using a CDN or npm.
- The
flickity.pkgd.min.js
file is required to initialize the slider.
2️⃣ HTML Structure
- Two carousels:
- Main Carousel – Displays the larger images.
- Thumbnail Navigation – A separate carousel for small preview images.
3️⃣ CSS Styling
- Main carousel: 80% width and centered.
- Thumbnail navigation: Smaller thumbnails with lower opacity for unselected images.
- Selected thumbnail: Highlighted with full opacity and a border.
4️⃣ JavaScript Initialization
cellAlign: "center"
– Ensures the selected image is centered.contain: true
– Prevents extra white space around images.wrapAround: true
– Allows infinite looping of images.asNavFor: ".carousel-main"
– Links the thumbnail carousel with the main one.
6. Enhancing with Custom Features
Here are some additional ways to enhance the slider:
Auto-play Feature
Enable automatic sliding for the main carousel:
autoPlay: 3000 // Slides every 3 seconds
Lazy Loading for Performance Optimization
If you have many images, enable lazy loading:
lazyLoad: true
Adding Next/Previous Buttons
If you want navigation buttons:
prevNextButtons: true
Final Thoughts
Using Flickity with thumbnail navigation is a great way to enhance user experience, especially for galleries and product showcases. The asNavFor
feature makes it easy to synchronize the main carousel with a thumbnail navigation bar.
Would you like help with additional customizations, such as captions, animations, or responsiveness?
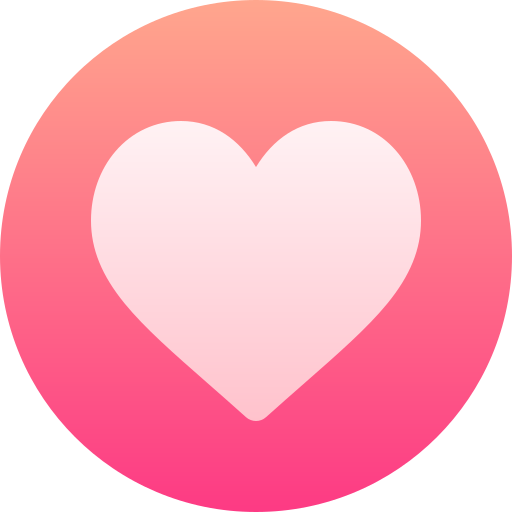