Steps to Convert a Website into an iOS App Using Android Studio (Flutter)
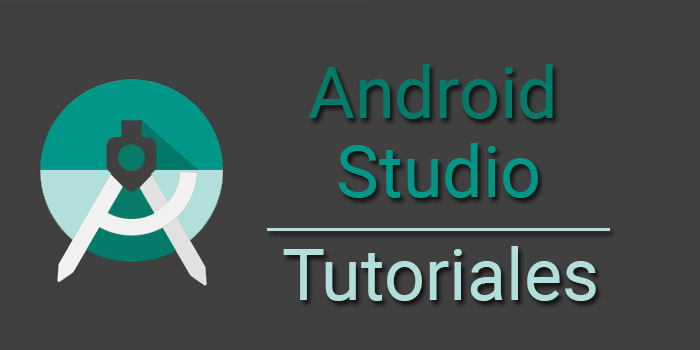
Converting a website into an iOS application using Android Studio is not a common approach, as Android Studio is primarily for Android development. However, you can use Flutter within Android Studio to create a cross-platform mobile app (iOS & Android). Below is a step-by-step guide to achieving this, including essential features like splash screens, progress bars, offline support, push notifications, and publishing.
To develop an iOS app from a website, we will use Flutter (a cross-platform framework) in Android Studio.
1. Install Required Tools
- Android Studio (with Flutter plugin)
- Xcode (for iOS development)
- Flutter SDK (https://flutter.dev)
- CocoaPods (for iOS dependencies, install via
sudo gem install cocoapods
)
2. Create a Flutter Project
- Open Android Studio → Click on "Start a new Flutter project".
- Select Flutter Application → Set a project name and location.
- Choose a development language (Dart is the default).
- Click Finish.
3. Add a WebView to Load Your Website
To display your website inside the app, use webview_flutter:
Install WebView Plugin
In pubspec.yaml
, add:
dependencies:
flutter:
sdk: flutter
webview_flutter: ^4.2.2
Run:
flutter pub get
Modify main.dart
to Use WebView
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: WebScreen(),
);
}
}
class WebScreen extends StatefulWidget {
@override
_WebScreenState createState() => _WebScreenState();
}
class _WebScreenState extends State<WebScreen> {
late WebViewController controller;
@override
Widget build(BuildContext context) {
return Scaffold(
body: WebView(
initialUrl: "https://yourwebsite.com",
javascriptMode: JavascriptMode.unrestricted,
onWebViewCreated: (WebViewController webViewController) {
controller = webViewController;
},
),
);
}
}
4. Add a Splash Screen
To create a splash screen, use the flutter_native_splash
package.
Install the Package
In pubspec.yaml
, add:
dev_dependencies:
flutter_native_splash: ^2.3.2
Run:
flutter pub get
Configure Splash Screen
Edit flutter_native_splash.yaml
:
flutter_native_splash:
color: "#ffffff"
image: assets/splash.png
android: true
ios: true
Run:
flutter pub run flutter_native_splash:create
5. Add a Progress Bar (Loading Indicator)
Modify WebScreen
to show a progress bar while loading:
class _WebScreenState extends State<WebScreen> {
late WebViewController controller;
bool isLoading = true;
@override
Widget build(BuildContext context) {
return Stack(
children: [
WebView(
initialUrl: "https://yourwebsite.com",
javascriptMode: JavascriptMode.unrestricted,
onWebViewCreated: (controller) {
this.controller = controller;
},
onPageFinished: (_) {
setState(() {
isLoading = false;
});
},
),
if (isLoading)
Center(child: CircularProgressIndicator()), // Show loader
],
);
}
}
6. Add Offline Support
To show a message when offline, use connectivity_plus
:
Install Package
In pubspec.yaml
, add:
dependencies:
connectivity_plus: ^5.0.2
Run:
flutter pub get
Modify WebScreen
to Check Connectivity
import 'package:connectivity_plus/connectivity_plus.dart';
@override
void initState() {
super.initState();
Connectivity().onConnectivityChanged.listen((ConnectivityResult result) {
if (result == ConnectivityResult.none) {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text('No Internet Connection')),
);
}
});
}
7. Implement Push Notifications
Use Firebase Cloud Messaging (FCM) for push notifications.
Steps:
-
Set up Firebase:
- Go to Firebase Console
- Create a project and add iOS
- Download the
GoogleService-Info.plist
and add it to your project.
-
Install Firebase Messaging Package
dependencies: firebase_messaging: ^14.6.2 firebase_core: ^2.14.0
-
Initialize Firebase in
main.dart
import 'package:firebase_core/firebase_core.dart'; import 'package:firebase_messaging/firebase_messaging.dart'; void main() async { WidgetsFlutterBinding.ensureInitialized(); await Firebase.initializeApp(); FirebaseMessaging messaging = FirebaseMessaging.instance; String? token = await messaging.getToken(); print("FCM Token: $token"); runApp(MyApp()); }
8. Publish to the App Store
Prerequisites
- Apple Developer Account ($99/year)
- A Mac computer with Xcode
- An app icon (1024x1024 PNG)
Steps to Publish
-
Prepare the App
flutter build ios
-
Open in Xcode
open ios/Runner.xcworkspace
-
Set Bundle ID & Team
- Go to Signing & Capabilities
- Choose a unique Bundle Identifier
- Select Development Team
-
Archive and Upload
- In Xcode, go to Product → Archive
- Click Distribute App → App Store Connect
- Submit for review.
Conclusion
You’ve successfully converted a website into an iOS app using Flutter in Android Studio. It includes: ✅ WebView
✅ Splash Screen
✅ Progress Bar
✅ Offline Support
✅ Push Notifications
✅ Publishing to App Store