Creating a Responsive Navbar with Advanced Features
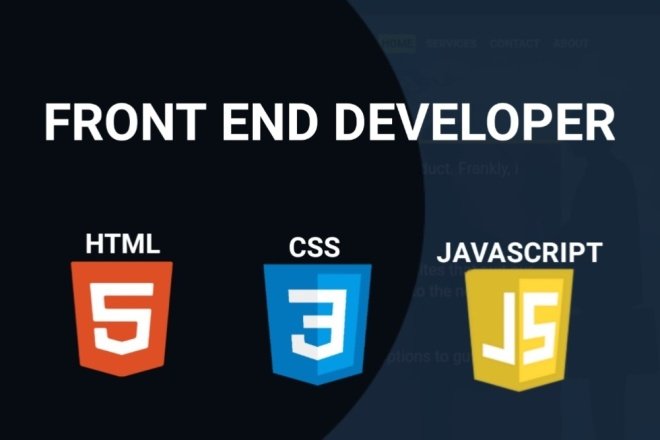
This guide will show you how to build a responsive navbar using HTML, CSS, and JavaScript with the following features:
✅ Sticky Navbar – Navbar remains fixed at the top when scrolling.
✅ Submenus – Dropdown menus for better navigation.
✅ Animations – Smooth transitions for an elegant look.
✅ Dark Mode Toggle – Switch between light and dark themes.
✅ Mobile-Friendly – A hamburger menu for small screens.
✅ Extra Features – Hover effects, icon animations, and theme memory.
Step 1: HTML Structure
The HTML will include:
- A logo.
- Main navigation menu with a submenu.
- Hamburger menu for mobile.
- A dark mode toggle button.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Advanced Responsive Navbar</title>
<link rel="stylesheet" href="styles.css">
<script defer src="script.js"></script>
</head>
<body>
<!-- Navigation Bar -->
<nav class="navbar">
<div class="logo">MyBrand</div>
<ul class="nav-links">
<li><a href="#">Home</a></li>
<li class="dropdown">
<a href="#">Services ▾</a>
<ul class="submenu">
<li><a href="#">Web Design</a></li>
<li><a href="#">App Development</a></li>
<li><a href="#">SEO Optimization</a></li>
</ul>
</li>
<li><a href="#">Portfolio</a></li>
<li><a href="#">Contact</a></li>
<li>
<button class="dark-mode-toggle">🌙</button>
</li>
</ul>
<div class="hamburger">
<span></span>
<span></span>
<span></span>
</div>
</nav>
<!-- Placeholder content to demonstrate sticky navbar -->
<section style="height: 2000px; padding: 20px;">
<h1>Scroll Down to See the Sticky Navbar</h1>
</section>
</body>
</html>
Step 2: CSS Styling
Now, let’s style the navbar, submenu, animations, dark mode, and sticky behavior.
/* styles.css */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: Arial, sans-serif;
}
/* Default Light Mode */
body {
background: white;
color: black;
transition: background 0.3s ease, color 0.3s ease;
}
/* Sticky Navbar */
.navbar {
display: flex;
justify-content: space-between;
align-items: center;
background-color: #333;
padding: 15px 20px;
color: white;
position: fixed;
top: 0;
width: 100%;
z-index: 1000;
transition: background 0.3s ease, padding 0.3s ease;
}
/* Navbar Shrink on Scroll */
.navbar.scrolled {
background: #222;
padding: 10px 20px;
}
/* Logo */
.logo {
font-size: 24px;
font-weight: bold;
}
/* Navigation Links */
.nav-links {
list-style: none;
display: flex;
align-items: center;
}
.nav-links li {
position: relative;
margin: 0 15px;
}
.nav-links a {
text-decoration: none;
color: white;
font-size: 18px;
transition: color 0.3s ease;
}
.nav-links a:hover {
color: #f4a261;
}
/* Submenu */
.submenu {
display: none;
position: absolute;
top: 100%;
left: 0;
background: #444;
list-style: none;
padding: 10px 0;
width: 200px;
border-radius: 5px;
opacity: 0;
transition: opacity 0.3s ease, transform 0.3s ease;
transform: translateY(10px);
}
.submenu li {
padding: 10px;
}
.submenu a {
color: white;
display: block;
}
.submenu a:hover {
background: #555;
}
/* Show Submenu on Hover */
.dropdown:hover .submenu {
display: block;
opacity: 1;
transform: translateY(0);
}
/* Hamburger Menu */
.hamburger {
display: none;
flex-direction: column;
cursor: pointer;
}
.hamburger span {
width: 30px;
height: 3px;
background: white;
margin: 5px 0;
transition: transform 0.3s ease;
}
/* Responsive Design */
@media (max-width: 768px) {
.nav-links {
display: none;
flex-direction: column;
position: absolute;
top: 60px;
left: 0;
width: 100%;
background: #333;
padding: 10px 0;
text-align: center;
}
.nav-links.active {
display: flex;
}
.hamburger {
display: flex;
}
/* Rotate Hamburger Icon */
.hamburger.active span:nth-child(1) {
transform: translateY(8px) rotate(45deg);
}
.hamburger.active span:nth-child(2) {
opacity: 0;
}
.hamburger.active span:nth-child(3) {
transform: translateY(-8px) rotate(-45deg);
}
}
/* Dark Mode */
.dark-mode {
background: black;
color: white;
}
.dark-mode .navbar {
background: #222;
}
.dark-mode .submenu {
background: #333;
}
.dark-mode .nav-links a {
color: white;
}
.dark-mode-toggle {
background: none;
border: none;
font-size: 18px;
cursor: pointer;
color: white;
}
Step 3: JavaScript for Interactivity
Now, let's add JavaScript for:
- Sticky navbar effect
- Mobile menu toggle
- Dark mode toggle with memory
// script.js
document.addEventListener("DOMContentLoaded", function () {
const navbar = document.querySelector(".navbar");
const hamburger = document.querySelector(".hamburger");
const navLinks = document.querySelector(".nav-links");
const darkModeToggle = document.querySelector(".dark-mode-toggle");
// Sticky Navbar Effect
window.addEventListener("scroll", () => {
if (window.scrollY > 50) {
navbar.classList.add("scrolled");
} else {
navbar.classList.remove("scrolled");
}
});
// Toggle Mobile Menu
hamburger.addEventListener("click", () => {
navLinks.classList.toggle("active");
hamburger.classList.toggle("active");
});
// Dark Mode Toggle
darkModeToggle.addEventListener("click", () => {
document.body.classList.toggle("dark-mode");
// Store user preference
if (document.body.classList.contains("dark-mode")) {
localStorage.setItem("theme", "dark");
} else {
localStorage.setItem("theme", "light");
}
});
// Load Theme Preference
if (localStorage.getItem("theme") === "dark") {
document.body.classList.add("dark-mode");
}
});
Final Features
✅ Sticky Navbar with Shrinking Effect
✅ Hamburger Menu for Mobile
✅ Dropdown Submenus with Animation
✅ Dark Mode Toggle with Local Storage
✅ Smooth Transitions & Advanced Animations
Would you like additional enhancements, such as scroll indicators, animated icons, or extra UI components?