How To Create a Responsive iFrame In HTML
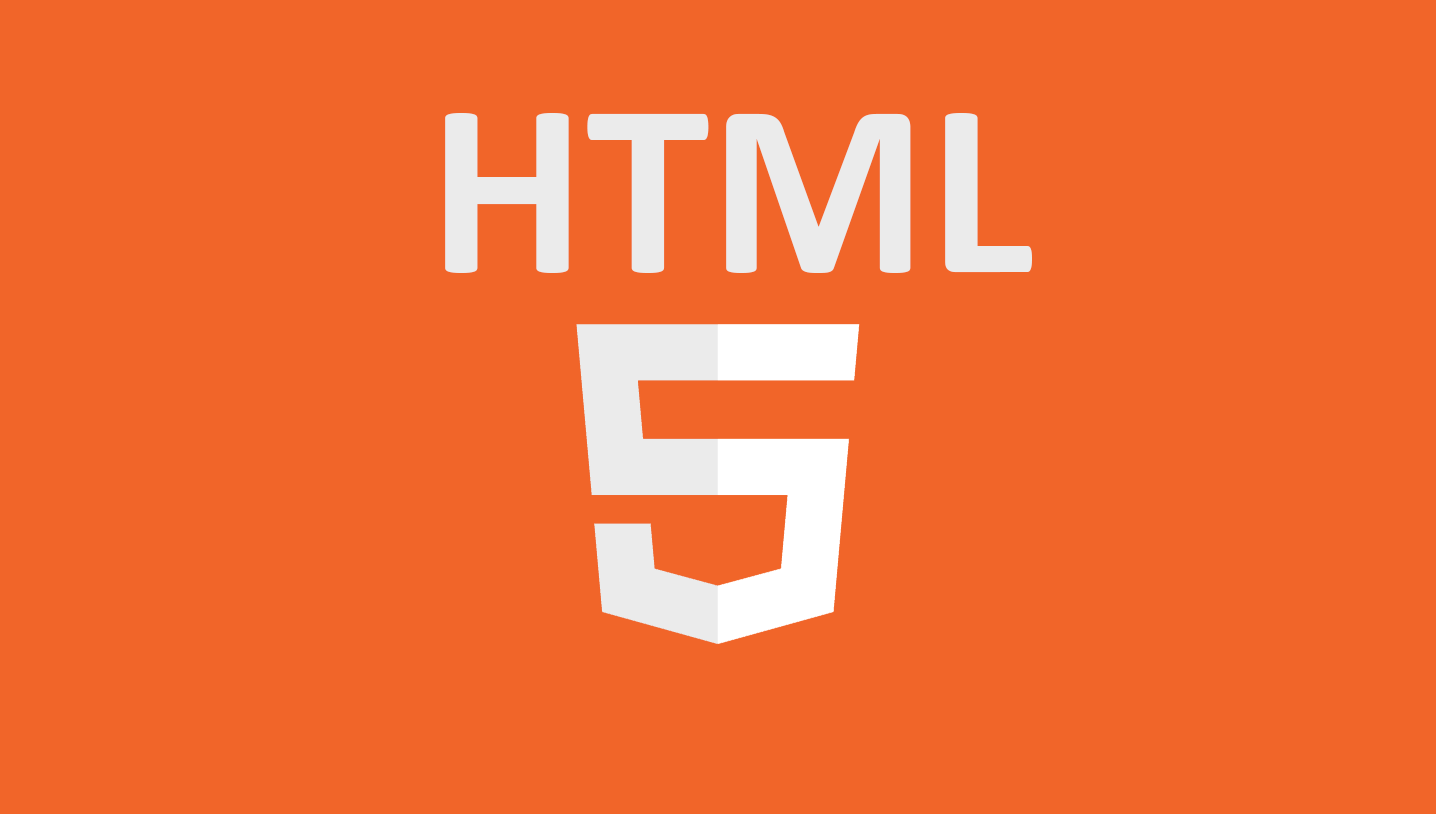
Advanced usage of HTML <iframe>
involves various techniques to enhance functionality, security, and user experience. Below are key advanced topics with examples:
🔹 1. Responsive iFrame (Auto Adjust Height)
Problem: Fixed-size iframes can cause scrolling issues.
Solution: Use JavaScript to adjust the height dynamically.
✅ Example: Auto-Adjusting iFrame Height
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive iFrame</title>
<style>
iframe {
width: 100%;
border: none;
}
</style>
</head>
<body>
<iframe id="myIframe" src="https://example.com"></iframe>
<script>
function resizeIframe() {
var iframe = document.getElementById("myIframe");
iframe.style.height = iframe.contentWindow.document.body.scrollHeight + "px";
}
document.getElementById("myIframe").onload = resizeIframe;
</script>
</body>
</html>
🔹 What it does: Dynamically resizes the <iframe>
height based on the content inside.
🔹 2. Passing Data Between Parent and iFrame
You can use postMessage()
to securely send data between the parent window and the iframe.
✅ Example: Sending Data from Parent to iFrame
Parent Page (index.html)
<!DOCTYPE html>
<html>
<head>
<title>Parent Page</title>
</head>
<body>
<iframe id="childFrame" src="child.html" width="600" height="400"></iframe>
<button onclick="sendMessage()">Send Message</button>
<script>
function sendMessage() {
var iframe = document.getElementById('childFrame').contentWindow;
iframe.postMessage("Hello from Parent!", "*"); // "*" allows communication with any domain
}
</script>
</body>
</html>
Child Page (child.html)
<!DOCTYPE html>
<html>
<head>
<title>Child Page</title>
</head>
<body>
<p>Waiting for a message...</p>
<script>
window.addEventListener("message", function(event) {
document.body.innerHTML += "<p>Received: " + event.data + "</p>";
});
</script>
</body>
</html>
🔹 What it does: Sends a message from the parent to the iframe and updates the iframe's content.
🔹 3. Allowing Full-Screen Mode in iFrame
By default, iframes do not allow fullscreen mode. You can enable it using the allowfullscreen
attribute.
✅ Example: Enabling Fullscreen iFrame
<iframe src="https://www.youtube.com/embed/dQw4w9WgXcQ" width="800" height="450" allowfullscreen></iframe>
🔹 What it does: Allows users to expand the iframe content into full screen.
🔹 4. Loading an iFrame Only When Visible (Lazy Loading)
Lazy loading improves performance by loading the iframe only when needed.
✅ Example: Lazy Load iFrame
<iframe src="https://example.com" width="600" height="400" loading="lazy"></iframe>
🔹 What it does: Prevents the iframe from loading until it appears on the screen.
🔹 5. Restricting Permissions in iFrame (Security)
The sandbox
attribute restricts iframe permissions to prevent security risks.
✅ Example: Safe Embedding with Sandbox
<iframe src="https://example.com" width="600" height="400" sandbox="allow-scripts allow-same-origin"></iframe>
🔹 What it does: Limits what the iframe can do, blocking actions like pop-ups, form submissions, and more.
🔹 6. Blocking iFrame from Being Embedded (Prevent Clickjacking)
To prevent your website from being embedded inside an iframe (to avoid clickjacking attacks), use X-Frame-Options
in the HTTP header.
✅ Example: Prevent Embedding Using HTTP Headers (Apache)
Add this to your .htaccess
file:
Header always set X-Frame-Options "SAMEORIGIN"
🔹 What it does: Blocks other sites from embedding your webpage inside an <iframe>
.
🔹 7. Display Different Content If iFrames Are Blocked
If a browser blocks iframes (e.g., due to security settings), provide alternative content.
✅ Example: Fallback Content
<iframe src="https://example.com" width="600" height="400">
<p>Your browser does not support iframes. <a href="https://example.com">Click here</a> to visit the page.</p>
</iframe>
🔹 What it does: Shows alternative content if iframes are blocked.
🔹 8. Customizing Scrollbars Inside iFrames
You can hide or style the iframe’s scrollbars using CSS.
✅ Example: Hide Scrollbars
<iframe src="https://example.com" width="600" height="400" style="overflow:hidden;"></iframe>
🔹 What it does: Hides scrollbars in supported browsers.
Conclusion
These advanced iframe techniques can enhance functionality, improve security, and optimize performance. Which one do you need help implementing?
- Artificial Intelligence (AI)
- Cybersecurity
- Blockchain & Cryptocurrencies
- Internet of Things
- Cloud Computing
- Big Data & Analytics
- Virtual Reality
- 5G & Future Connectivity
- Robotics & Automation
- Software Development & Programming
- Tech Hardware & Devices
- Tech in Healthcare
- Tech in Business
- Gaming Technologies
- Tech in Education
- Machine Learning (ML)
- Blogging
- Affiliate Marketing
- Make Money
- Digital Marketing
- Product Review
- Social Media
- Excel
- Graphics design
- Freelancing/Consulting
- FinTech (Financial Technology)
- E-commerce and Digital Marketing
- Business
- Sport
- Self Development
- Tips to Success
- Video Editing
- Photo Editing
- Website Promotion
- YouTube
- Lifestyle
- Health
- Computer
- Phone
- Music
- Accounting
- Causes
- Networking