The Basics of Python to get started
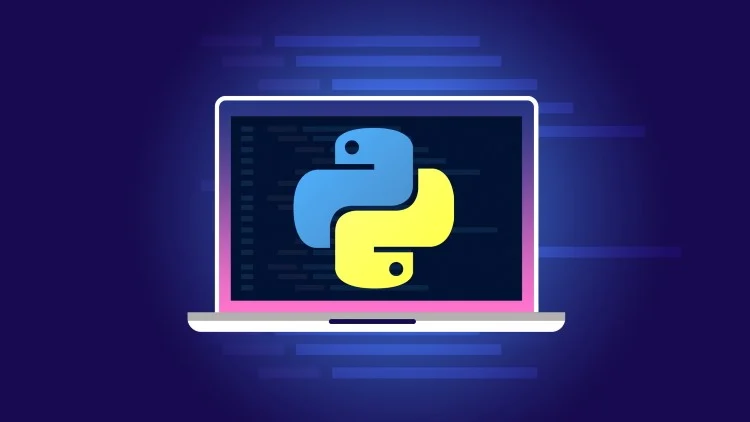
Basics of Python
1. Introduction to Python
Python is a high-level, interpreted programming language known for its simplicity and readability. It was created by Guido van Rossum and first released in 1991. Python is widely used for web development, data science, automation, artificial intelligence, and more. Its easy-to-learn syntax makes it a great choice for beginners.
2. Installing Python & Setting Up Environment
To start using Python, follow these steps:
- Download and Install: Visit python.org and download the latest version for your OS.
- Verify Installation: Open a terminal or command prompt and type:
orpython --version
python3 --version
- Using an IDE: You can write Python code using:
- IDLE (comes with Python)
- VS Code
- PyCharm
- Jupyter Notebook (for data science)
3. Python Syntax and Indentation
Python syntax is clean and uses indentation (whitespace) to define blocks of code instead of curly braces {}
.
Example:
if 5 > 2:
print("Five is greater than two") # Indentation is mandatory
Incorrect indentation will result in an IndentationError.
4. Variables and Data Types
Variables in Python are dynamically typed, meaning you don’t need to declare their type explicitly.
x = 10 # Integer
y = 3.14 # Float
name = "Python" # String
is_valid = True # Boolean
fruits = ["Apple", "Banana", "Cherry"] # List
5. Type Conversion
Python allows you to convert one data type into another.
a = 5
b = float(a) # Converts integer to float
c = str(a) # Converts integer to string
d = int("10") # Converts string to integer
6. Operators
Python provides different types of operators:
-
Arithmetic Operators (
+
,-
,*
,/
,//
,%
,**
)a = 10 b = 3 print(a + b) # 13 print(a / b) # 3.333 print(a // b) # 3 (Floor division)
-
Comparison Operators (
==
,!=
,>
,<
,>=
,<=
)print(5 > 3) # True print(10 == 20) # False
-
Logical Operators (
and
,or
,not
)x = True y = False print(x and y) # False print(x or y) # True print(not x) # False
-
Bitwise Operators (
&
,|
,^
,~
,<<
,>>
)a = 5 # 0101 in binary b = 3 # 0011 in binary print(a & b) # 0001 (1 in decimal) print(a | b) # 0111 (7 in decimal)
7. Input and Output
Python allows user input using input()
and output using print()
.
name = input("Enter your name: ")
print("Hello, " + name + "!")
This covers the basics of Python to get started! Let me know if you need further explanations.