Developing Smart Contracts and Creating a Cryptocurrency Wallet: A Detailed Guide
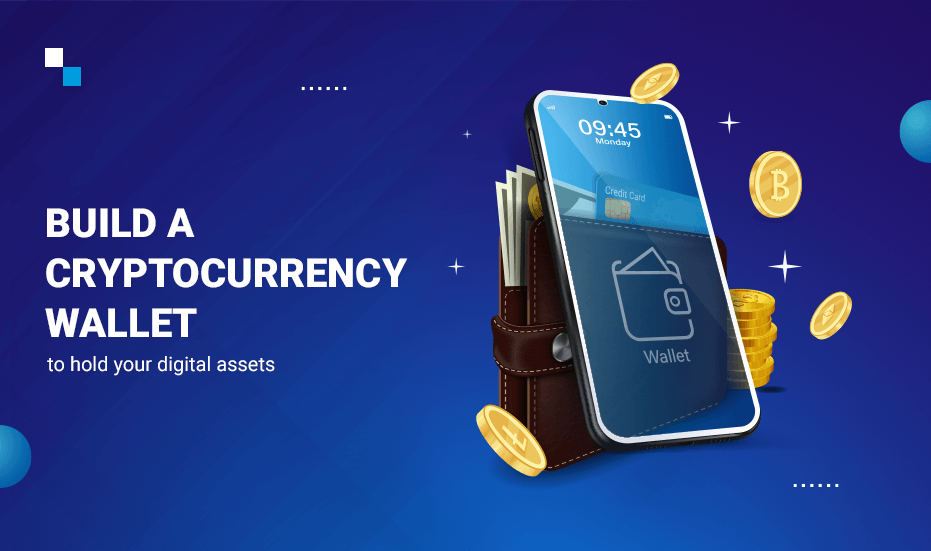
Blockchain technology has revolutionized the financial world, enabling decentralized applications (dApps) and smart contracts. This guide will walk you through developing smart contracts and creating a cryptocurrency wallet with full source code, explanations, key points, and best practices.
1. Developing Smart Contracts
A smart contract is a self-executing program stored on a blockchain that runs when predefined conditions are met.
Prerequisites
- Basic knowledge of Solidity (Ethereum’s smart contract language).
- Node.js and npm installed.
- Hardhat or Truffle for smart contract development.
- A crypto wallet like MetaMask for testing.
- Ganache (for local blockchain testing) or Remix IDE (web-based).
Step 1: Setting Up the Development Environment
-
Install Node.js and npm:
node -v npm -v
If not installed, download from Node.js official site.
-
Install Hardhat:
npm install --save-dev hardhat
Initialize a project:
npx hardhat
Step 2: Writing a Smart Contract
Create a Solidity file SimpleToken.sol
inside the contracts
directory.
SimpleToken.sol (Basic ERC-20 Token)
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract SimpleToken is ERC20 {
constructor(uint256 initialSupply) ERC20("SimpleToken", "STK") {
_mint(msg.sender, initialSupply * (10 ** decimals()));
}
}
Key Points
@openzeppelin/contracts/token/ERC20/ERC20.sol
provides a secure ERC-20 implementation.ERC20("SimpleToken", "STK")
sets the token name and symbol._mint(msg.sender, initialSupply * (10 ** decimals()))
creates initial supply.
Step 3: Deploying the Smart Contract
-
Configure Hardhat (
hardhat.config.js
):require("@nomicfoundation/hardhat-toolbox"); module.exports = { solidity: "0.8.0", networks: { hardhat: {}, rinkeby: { url: "https://rinkeby.infura.io/v3/YOUR_INFURA_PROJECT_ID", accounts: ["YOUR_PRIVATE_KEY"] } } };
Replace
YOUR_INFURA_PROJECT_ID
andYOUR_PRIVATE_KEY
. -
Create a deployment script (
scripts/deploy.js
):const hre = require("hardhat"); async function main() { const Token = await hre.ethers.getContractFactory("SimpleToken"); const token = await Token.deploy(1000000); await token.deployed(); console.log("Token deployed to:", token.address); } main().catch((error) => { console.error(error); process.exit(1); });
-
Run the deployment:
npx hardhat run scripts/deploy.js --network rinkeby
Key Points
- Infura API enables blockchain interactions.
deploy()
handles contract creation and deployment.- Rinkeby testnet is used for testing before mainnet deployment.
2. Creating a Cryptocurrency Wallet
A cryptocurrency wallet allows users to send, receive, and manage digital assets.
Prerequisites
- React.js for frontend.
- ethers.js for Ethereum interactions.
- MetaMask for wallet integration.
Step 1: Setting Up the React Project
-
Install React:
npx create-react-app crypto-wallet cd crypto-wallet
-
Install dependencies:
npm install ethers web3 react-toastify
Step 2: Implementing Wallet Functions
Modify App.js
:
import React, { useState } from "react";
import { ethers } from "ethers";
function App() {
const [account, setAccount] = useState(null);
const [balance, setBalance] = useState(null);
const connectWallet = async () => {
if (window.ethereum) {
const provider = new ethers.providers.Web3Provider(window.ethereum);
await window.ethereum.request({ method: "eth_requestAccounts" });
const signer = provider.getSigner();
const address = await signer.getAddress();
const balance = await provider.getBalance(address);
setAccount(address);
setBalance(ethers.utils.formatEther(balance));
} else {
alert("MetaMask is required.");
}
};
return (
<div>
<h1>Crypto Wallet</h1>
<button onClick={connectWallet}>Connect Wallet</button>
{account && (
<div>
<p>Account: {account}</p>
<p>Balance: {balance} ETH</p>
</div>
)}
</div>
);
}
export default App;
Key Points
window.ethereum.request({ method: "eth_requestAccounts" })
connects MetaMask.ethers.providers.Web3Provider
fetches blockchain data.ethers.utils.formatEther(balance)
converts Wei to Ether.
Step 3: Sending Transactions
Modify App.js
:
const sendTransaction = async () => {
if (!account) return alert("Connect your wallet first!");
const provider = new ethers.providers.Web3Provider(window.ethereum);
const signer = provider.getSigner();
try {
const tx = await signer.sendTransaction({
to: "0xReceiverAddress", // Replace with recipient
value: ethers.utils.parseEther("0.01") // Send 0.01 ETH
});
await tx.wait();
alert(`Transaction successful: ${tx.hash}`);
} catch (error) {
alert("Transaction failed: " + error.message);
}
};
Add a button:
<button onClick={sendTransaction}>Send 0.01 ETH</button>
Key Points
signer.sendTransaction()
sends ETH.tx.wait()
confirms the transaction.- Error handling ensures robustness.
Final Thoughts
Smart Contracts
✔ Secure and self-executing
✔ Used in DeFi, NFTs, DAOs
✔ Requires testing before deployment
Crypto Wallet
✔ Stores and transfers tokens
✔ Uses MetaMask & ethers.js
✔ Essential for dApp interactions
This guide gives you a solid foundation in smart contract development and wallet creation. Ready to explore DeFi, NFTs, or custom blockchain solutions?