Steps on how to Connect HTML to PHP
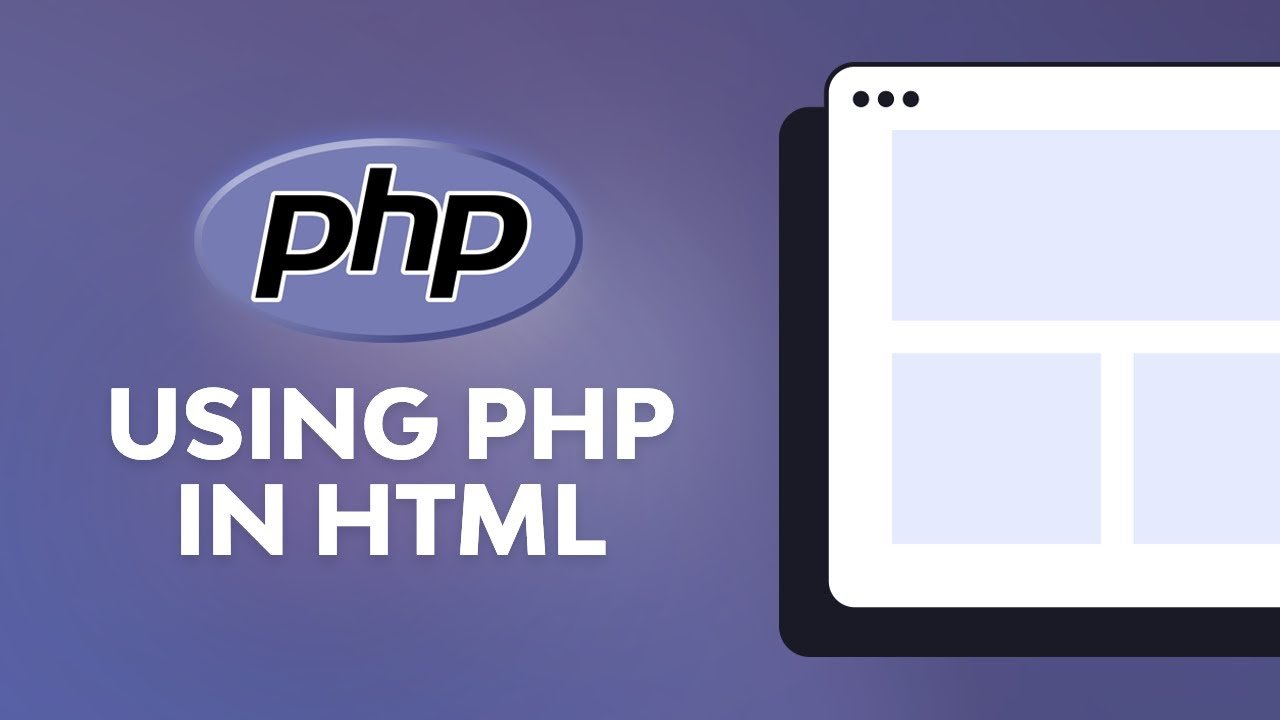
To connect HTML to PHP, you'll typically be using a .php
file for the server-side code, while your HTML can either be embedded inside the PHP file or linked to it. Here’s a basic guide on how to do that:
1. Basic Setup
First, ensure you have a server environment that supports PHP. You can use local servers like XAMPP, MAMP, or WAMP, or host your files on a live server that supports PHP.
2. Embedding PHP in HTML
PHP can be embedded directly into an HTML document using the <?php ... ?>
tags. When the PHP file is accessed, the PHP code will run on the server, and the result will be sent to the browser as regular HTML.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>PHP with HTML</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<p>
Today’s date is:
<?php
// PHP code to get the current date
echo date('Y-m-d');
?>
</p>
<form action="process.php" method="POST">
<label for="name">Enter your name:</label>
<input type="text" id="name" name="name">
<input type="submit" value="Submit">
</form>
</body>
</html>
In this example:
- The PHP code inside the
<p>
tag displays the current date. - There's also a simple form that sends data (like a name) to another PHP file (
process.php
) using the POST method.
3. Separating PHP and HTML
If you want to keep your PHP and HTML files separated for organization, you can:
- Create a
.php
file for your logic (e.g.,process.php
). - Use an HTML form to send data to the PHP file for processing.
Example:
<!-- index.html (or index.php) -->
<form action="process.php" method="POST">
<input type="text" name="name" placeholder="Enter your name">
<input type="submit" value="Submit">
</form>
<!-- process.php -->
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = $_POST['name'];
echo "Hello, " . htmlspecialchars($name) . "!";
}
?>
In this case, the HTML form sends data to process.php
, where PHP processes it and outputs a greeting.
Key Points:
- The file should be saved with a
.php
extension, not.html
, for the server to process the PHP code. - The form action (e.g.,
action="process.php"
) specifies which PHP file will handle the submitted data. - PHP can generate dynamic content and interact with HTML using embedded PHP code.
Let me know if you need more examples or if you have any questions!