What to do When working with the DOM
Posted 2025-02-03 17:34:36
0
698
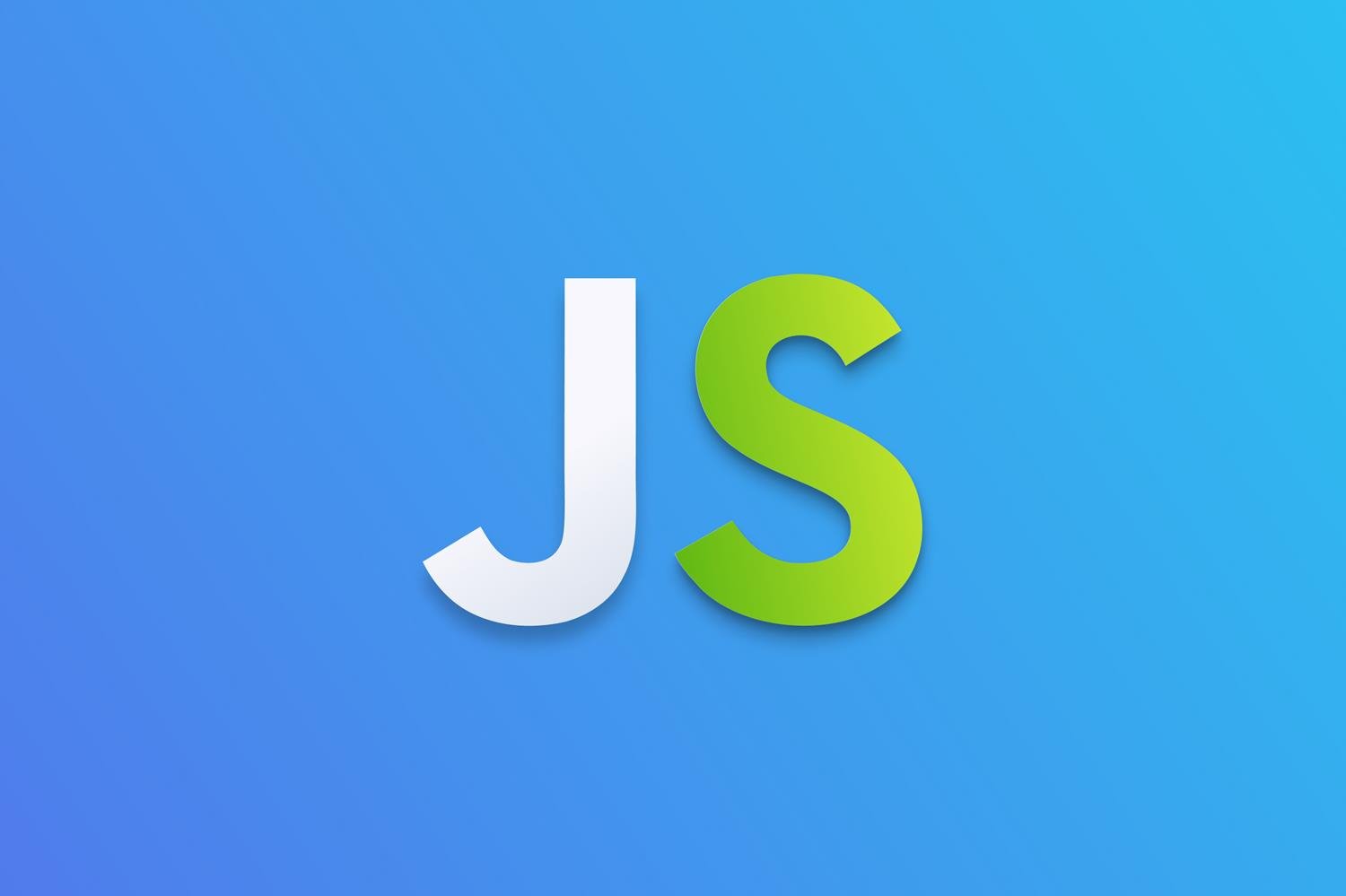
When working with the DOM (Document Object Model) in JavaScript, you're essentially interacting with the structure of an HTML document. Here are some key concepts and methods you might find useful:
1. Selecting Elements
getElementById(id)
: Selects an element with a specific ID.let element = document.getElementById('myId');
getElementsByClassName(className)
: Selects all elements with a specific class name.let elements = document.getElementsByClassName('myClass');
getElementsByTagName(tagName)
: Selects all elements with a specific tag name.let elements = document.getElementsByTagName('div');
querySelector(selector)
: Selects the first element that matches a CSS selector.let element = document.querySelector('.myClass');
querySelectorAll(selector)
: Selects all elements that match a CSS selector.let elements = document.querySelectorAll('div.myClass');
2. Manipulating Elements
- Changing Content:
element.textContent = 'New content'; element.innerHTML = '<p>New HTML content</p>';
- Changing Styles:
element.style.color = 'red'; element.style.backgroundColor = 'blue';
- Adding/Removing Classes:
element.classList.add('newClass'); element.classList.remove('oldClass'); element.classList.toggle('toggleClass');
- Setting Attributes:
element.setAttribute('src', 'image.jpg'); element.setAttribute('alt', 'Description');
- Removing Elements:
element.remove();
3. Event Handling
- Adding Event Listeners:
element.addEventListener('click', function() { alert('Element clicked!'); });
- Removing Event Listeners:
function handleClick() { alert('Element clicked!'); } element.addEventListener('click', handleClick); element.removeEventListener('click', handleClick);
4. Creating and Appending Elements
- Creating Elements:
let newElement = document.createElement('div'); newElement.textContent = 'Hello, World!';
- Appending Elements:
document.body.appendChild(newElement);
5. Traversing the DOM
- Parent Node:
let parent = element.parentNode;
- Child Nodes:
let children = element.childNodes;
- Sibling Nodes:
let nextSibling = element.nextSibling; let previousSibling = element.previousSibling;
Feel free to ask if you need more details on any of these topics!
Search
Sellect from all Categories bellow ⇓
- Artificial Intelligence (AI)
- Cybersecurity
- Blockchain & Cryptocurrencies
- Internet of Things
- Cloud Computing
- Big Data & Analytics
- Virtual Reality
- 5G & Future Connectivity
- Robotics & Automation
- Software Development & Programming
- Tech Hardware & Devices
- Tech in Healthcare
- Tech in Business
- Gaming Technologies
- Tech in Education
- Machine Learning (ML)
- Blogging
- Affiliate Marketing
- Make Money
- Digital Marketing
- Product Review
- Social Media
- Excel
- Graphics design
- Freelancing/Consulting
- FinTech (Financial Technology)
- E-commerce and Digital Marketing
- Business
- Sport
- Self Development
- Tips to Success
- Video Editing
- Photo Editing
- Website Promotion
- YouTube
- Lifestyle
- Health
- Computer
- Phone
- Music
- Accounting
- Causes
- Networking
Read More
Steps On How to Analyze Cryptocurrencies Before Investing
Investing in cryptocurrencies can be lucrative,...
Introduction to HTML
1. Description of HTML
HTML (HyperText Markup...
How to Build a Website with UI/UX Design in Mind
1. Understand the Purpose of the Website...
Tools To Use As an ethical hacker (also known as a white-hat hacker or penetration tester)
As an ethical hacker (also known as a white-hat...
How to Set up Node.js in Visual Studio Code
Setting up Node.js in Visual Studio Code is...