JavaScript form validation
Posted 2025-02-03 17:37:05
0
698
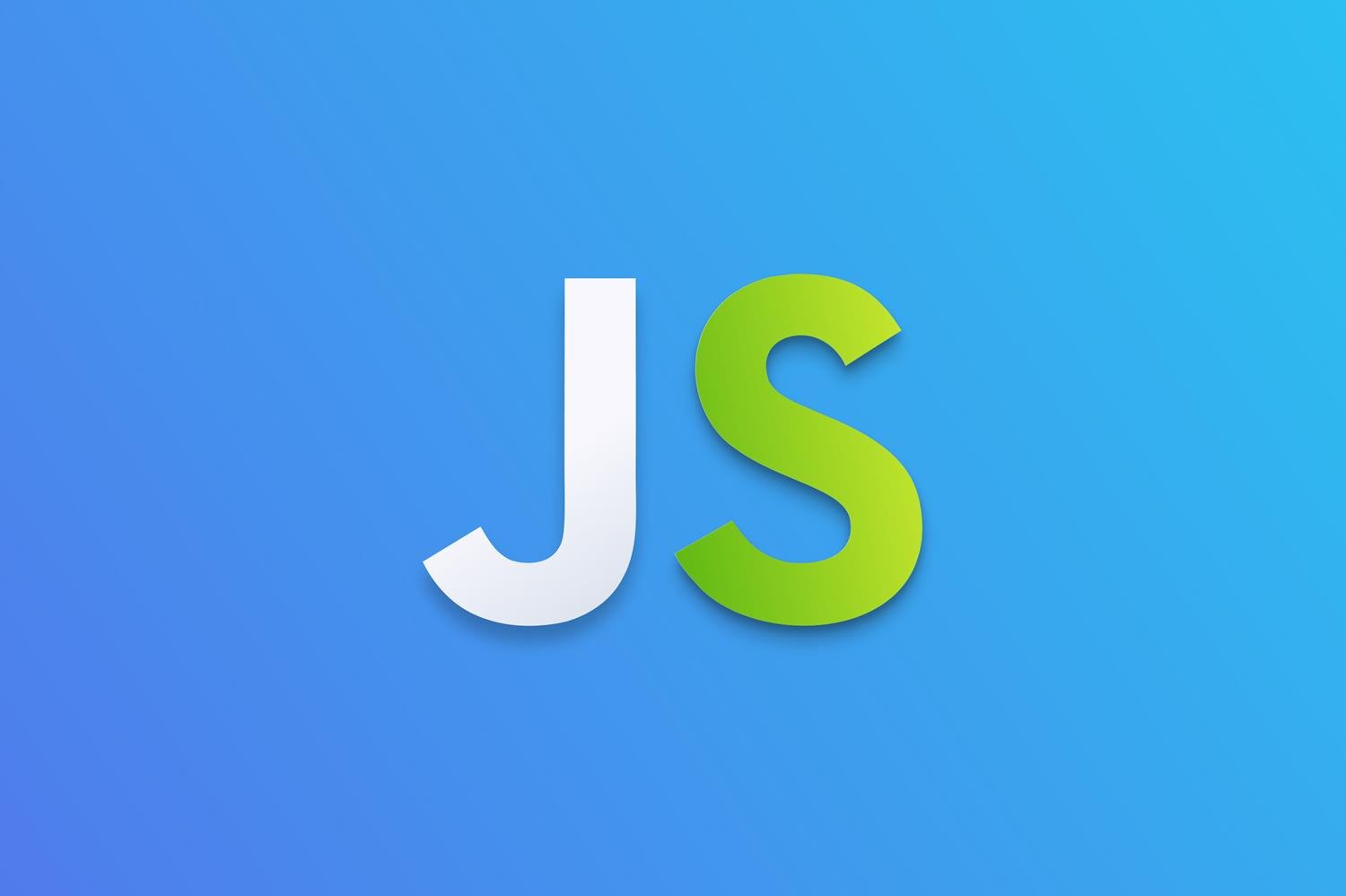
JavaScript form validation is a technique used to ensure that the data submitted in a form is correct and complete before it is sent to the server. Here's a basic example of how you can implement form validation using JavaScript:
HTML Form
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Form Validation</title>
<style>
.error {
color: red;
font-size: 0.875em;
}
</style>
</head>
<body>
<form id="myForm">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<span class="error" id="nameError"></span><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email">
<span class="error" id="emailError"></span><br><br>
<input type="submit" value="Submit">
</form>
<script src="form-validation.js"></script>
</body>
</html>
JavaScript Validation
Create a file named form-validation.js
with the following code:
document.getElementById('myForm').addEventListener('submit', function(event) {
let isValid = true;
// Clear previous errors
document.getElementById('nameError').textContent = '';
document.getElementById('emailError').textContent = '';
// Validate Name
const name = document.getElementById('name').value;
if (name.trim() === '') {
document.getElementById('nameError').textContent = 'Name is required';
isValid = false;
}
// Validate Email
const email = document.getElementById('email').value;
const emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!emailPattern.test(email)) {
document.getElementById('emailError').textContent = 'Invalid email format';
isValid = false;
}
// If form is invalid, prevent submission
if (!isValid) {
event.preventDefault();
}
});
Explanation
-
HTML Form:
- Contains fields for name and email.
<span>
elements are used to display error messages.
-
JavaScript:
- Adds an event listener to the form that listens for the
submit
event. - Clears any previous error messages.
- Validates the name field to ensure it's not empty.
- Validates the email field using a regular expression pattern to ensure it has the correct format.
- If validation fails, it prevents the form from being submitted by calling
event.preventDefault()
.
- Adds an event listener to the form that listens for the
You can expand this example by adding more fields and validations as needed.
Search
Sellect from all Categories bellow ⇓
- Artificial Intelligence (AI)
- Cybersecurity
- Blockchain & Cryptocurrencies
- Internet of Things
- Cloud Computing
- Big Data & Analytics
- Virtual Reality
- 5G & Future Connectivity
- Robotics & Automation
- Software Development & Programming
- Tech Hardware & Devices
- Tech in Healthcare
- Tech in Business
- Gaming Technologies
- Tech in Education
- Machine Learning (ML)
- Blogging
- Affiliate Marketing
- Make Money
- Digital Marketing
- Product Review
- Social Media
- Excel
- Graphics design
- Freelancing/Consulting
- FinTech (Financial Technology)
- E-commerce and Digital Marketing
- Business
- Sport
- Self Development
- Tips to Success
- Video Editing
- Photo Editing
- Website Promotion
- YouTube
- Lifestyle
- Health
- Computer
- Phone
- Music
- Accounting
- Causes
- Networking
Read More
Comprehensive Guide to HTML Attributes
HTML attributes provide additional information...
Introduction to Computer Science
Computer Science is a vast and dynamic field...
Steps to Create a custom WordPress blog theme
Creating a custom WordPress blog theme requires...
Meaning of Network security
Network security refers to the measures,...
How Smart Contracts Work: The Backbone of Decentralized Apps
Smart contracts are self-executing contracts...