Steps to Create a Flickity Slider with Custom Animations
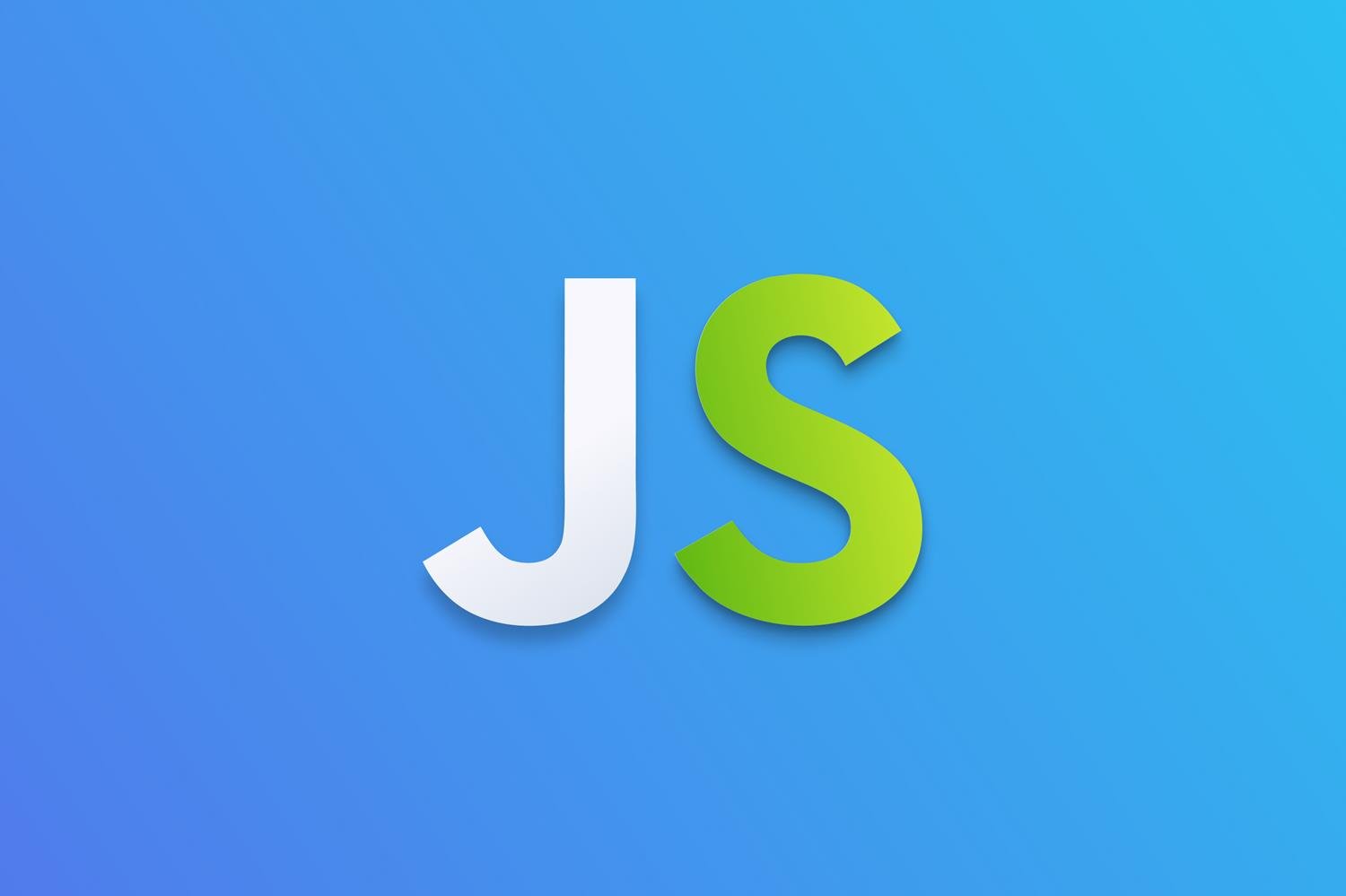
Creating a Flickity slider with custom animations in JavaScript involves integrating the Flickity carousel library and enhancing it with animations using CSS and JavaScript.
1. Include Flickity in Your Project
To use Flickity, you need to include the Flickity library via a CDN or npm.
CDN Method
Include the following in your HTML <head>
:
<!-- Flickity CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/flickity/2.2.2/flickity.min.css">
Place this before the closing <body>
tag:
<!-- Flickity JS -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/flickity/2.2.2/flickity.pkgd.min.js"></script>
NPM Method
If you're using npm, install Flickity:
npm install flickity
Then, import it into your JavaScript file:
import Flickity from 'flickity';
import 'flickity/css/flickity.css';
2. HTML Structure for the Slider
Create a simple slider with multiple slides.
<div class="carousel">
<div class="carousel-cell">1</div>
<div class="carousel-cell">2</div>
<div class="carousel-cell">3</div>
<div class="carousel-cell">4</div>
</div>
3. CSS for Styling & Animations
Style the slider and define animations.
.carousel {
background: #fafafa;
width: 80%;
margin: 0 auto;
}
.carousel-cell {
width: 300px;
height: 200px;
margin: 10px;
background: #ff5733;
color: white;
font-size: 2rem;
display: flex;
align-items: center;
justify-content: center;
border-radius: 10px;
transition: transform 0.4s ease-in-out, opacity 0.4s ease-in-out;
}
/* Custom animation for active slide */
.carousel-cell.is-selected {
transform: scale(1.2);
opacity: 1;
}
.carousel-cell:not(.is-selected) {
opacity: 0.5;
transform: scale(0.9);
}
Key Points in CSS
.carousel-cell.is-selected
: Applies a zoom effect to the active slide.- Opacity & Scale: Non-active slides have reduced opacity and are smaller.
4. JavaScript to Initialize Flickity with Custom Animations
Initialize Flickity and enhance it with custom animations.
document.addEventListener("DOMContentLoaded", function () {
let elem = document.querySelector('.carousel');
let flkty = new Flickity(elem, {
cellAlign: 'center',
contain: true,
wrapAround: true,
autoPlay: 3000,
pauseAutoPlayOnHover: false,
prevNextButtons: true,
pageDots: true
});
// Add custom animations on slide change
flkty.on('change', function (index) {
let cells = document.querySelectorAll('.carousel-cell');
cells.forEach((cell, i) => {
if (i === index) {
cell.classList.add("is-selected");
} else {
cell.classList.remove("is-selected");
}
});
});
});
5. Explanation of Key Points
✅ Using Flickity Initialization Options
cellAlign: 'center'
→ Centers slides.contain: true
→ Ensures all cells fit within the container.wrapAround: true
→ Allows infinite looping.autoPlay: 3000
→ Moves to the next slide every 3 seconds.pauseAutoPlayOnHover: false
→ Keeps autoplay running even when hovered.prevNextButtons: true
→ Enables navigation arrows.pageDots: true
→ Shows dot indicators.
✅ Handling Slide Change Events
- The
change
event triggers a function that applies/removes theis-selected
class. - This ensures that only the currently active slide gets the zoom effect.
6. Additional Enhancements
✨ Custom Fade Effect
If you want a fade effect between slides, you can use:
.carousel-cell {
opacity: 0;
transition: opacity 0.6s ease-in-out;
}
.carousel-cell.is-selected {
opacity: 1;
}
✨ GSAP Animation for a More Advanced Effect
For even more control, use GSAP for animations:
flkty.on('change', function (index) {
let cells = document.querySelectorAll('.carousel-cell');
gsap.to(cells, { scale: 0.9, opacity: 0.5, duration: 0.5 });
gsap.to(cells[index], { scale: 1.2, opacity: 1, duration: 0.5 });
});
Final Thoughts
By combining Flickity, CSS transitions, and JavaScript event handling, you can create a highly customized slider with animations. If you want even more complex effects, integrating GSAP can add smooth animations.