Method 2: Converting a Website into an Android App Using Android Studio
Posté 2025-02-21 11:24:00
0
395
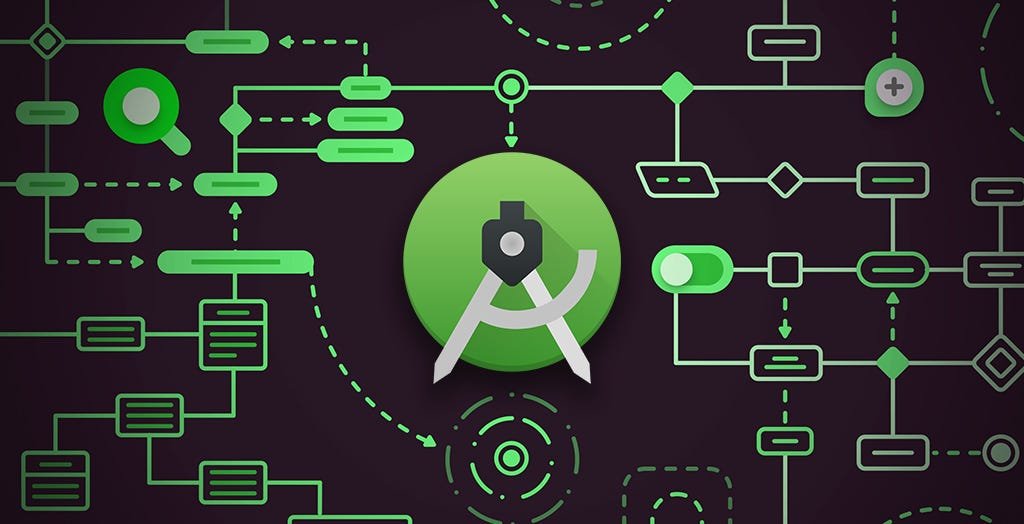
I'll provide a comprehensive step-by-step guide on converting a website into an Android app using Android Studio, including all the requested features. Let's get started.
Step 1: Set Up a New Android Studio Project
- Open Android Studio.
- Click on "Start a new Android Studio project".
- Select "Empty Activity" and click Next.
- Name your application (e.g.,
MyWebApp
). - Choose Java or Kotlin as the language.
- Click Finish to create your project.
Step 2: Add WebView to Load Website
Modify AndroidManifest.xml
- Open
AndroidManifest.xml
and add internet permission:<uses-permission android:name="android.permission.INTERNET"/>
- Add
WebView
to youractivity_main.xml
:<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <WebView android:id="@+id/webView" android:layout_width="match_parent" android:layout_height="match_parent" /> </androidx.constraintlayout.widget.ConstraintLayout>
Modify MainActivity.java
package com.example.mywebapp;
import android.annotation.SuppressLint;
import android.graphics.Bitmap;
import android.os.Bundle;
import android.view.View;
import android.webkit.WebChromeClient;
import android.webkit.WebResourceRequest;
import android.webkit.WebSettings;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import android.widget.ProgressBar;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private WebView webView;
private ProgressBar progressBar;
@SuppressLint("SetJavaScriptEnabled")
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
webView = findViewById(R.id.webView);
progressBar = findViewById(R.id.progressBar);
WebSettings webSettings = webView.getSettings();
webSettings.setJavaScriptEnabled(true);
webSettings.setDomStorageEnabled(true);
webView.setWebViewClient(new WebViewClient() {
@Override
public boolean shouldOverrideUrlLoading(WebView view, WebResourceRequest request) {
view.loadUrl(request.getUrl().toString());
return true;
}
@Override
public void onPageStarted(WebView view, String url, Bitmap favicon) {
progressBar.setVisibility(View.VISIBLE);
}
@Override
public void onPageFinished(WebView view, String url) {
progressBar.setVisibility(View.GONE);
}
});
webView.setWebChromeClient(new WebChromeClient());
webView.loadUrl("https://www.example.com"); // Replace with your website URL
}
@Override
public void onBackPressed() {
if (webView.canGoBack()) {
webView.goBack();
} else {
super.onBackPressed();
}
}
}
Step 3: Add Splash Screen
-
Create a new
SplashActivity.java
file:package com.example.mywebapp; import android.content.Intent; import android.os.Bundle; import android.os.Handler; import androidx.appcompat.app.AppCompatActivity; public class SplashActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_splash); new Handler().postDelayed(() -> { startActivity(new Intent(SplashActivity.this, MainActivity.class)); finish(); }, 3000); // 3-second delay } }
-
Create
activity_splash.xml
:<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:background="@color/white" android:orientation="vertical"> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/logo" /> </LinearLayout>
-
Set
SplashActivity
as the launcher inAndroidManifest.xml
:<activity android:name=".SplashActivity"> <intent-filter> <action android:name="android.intent.action.MAIN"/> <category android:name="android.intent.category.LAUNCHER"/> </intent-filter> </activity>
Step 4: Add Offline Support
Modify MainActivity.java
:
@Override
public void onReceivedError(WebView view, int errorCode, String description, String failingUrl) {
webView.loadUrl("file:///android_asset/offline.html");
}
Create offline.html
inside assets
folder:
<!DOCTYPE html>
<html>
<head>
<title>No Internet</title>
</head>
<body>
<h2>You are offline</h2>
<p>Please check your internet connection.</p>
</body>
</html>
Step 5: Add Push Notifications (Firebase)
- Add Firebase to your project from the Firebase Console.
- Add the
google-services.json
file to your app. - Modify
build.gradle (app)
:implementation 'com.google.firebase:firebase-messaging:23.0.0'
- Create
FirebaseMessagingService.java
:package com.example.mywebapp; import android.app.NotificationChannel; import android.app.NotificationManager; import android.os.Build; import androidx.core.app.NotificationCompat; import com.google.firebase.messaging.FirebaseMessagingService; import com.google.firebase.messaging.RemoteMessage; public class MyFirebaseMessagingService extends FirebaseMessagingService { @Override public void onMessageReceived(RemoteMessage remoteMessage) { NotificationManager notificationManager = (NotificationManager) getSystemService(NOTIFICATION_SERVICE); if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) { NotificationChannel channel = new NotificationChannel("default", "Default Channel", NotificationManager.IMPORTANCE_HIGH); notificationManager.createNotificationChannel(channel); } NotificationCompat.Builder notificationBuilder = new NotificationCompat.Builder(this, "default") .setContentTitle(remoteMessage.getNotification().getTitle()) .setContentText(remoteMessage.getNotification().getBody()) .setSmallIcon(R.drawable.ic_notification) .setAutoCancel(true); notificationManager.notify(1, notificationBuilder.build()); } }
Step 6: Customize Navigation and Status Bar
Modify styles.xml
:
<item name="android:statusBarColor">@color/black</item>
<item name="android:navigationBarColor">@color/black</item>
Step 7: Publishing to Google Play
- Generate Signed APK:
- Go to Build > Generate Signed Bundle/APK.
- Select APK, create a new keystore, and sign your app.
- Upload to Google Play Console:
- Create a developer account.
- Prepare a store listing (title, description, screenshots).
- Upload your signed APK.
- Set pricing, distribution, and content rating.
- Submit for review.
This guide provides a full working solution for converting your website into an Android app. Let me know if you need more details!
Rechercher
Sellect from all Catégories bellow ⇓
- Artificial Intelligence (AI)
- Cybersecurity
- Blockchain & Cryptocurrencies
- Internet of Things
- Cloud Computing
- Big Data & Analytics
- Virtual Reality
- 5G & Future Connectivity
- Robotics & Automation
- Software Development & Programming
- Tech Hardware & Devices
- Tech in Healthcare
- Tech in Business
- Gaming Technologies
- Tech in Education
- Machine Learning (ML)
- Blogging
- Affiliate Marketing
- Make Money
- Digital Marketing
- Product Review
- Social Media
- Excel
- Graphics design
- Freelancing/Consulting
- FinTech (Financial Technology)
- E-commerce and Digital Marketing
- Business
- Sport
- Self Development
- Tips to Success
- Video Editing
- Photo Editing
- Website Promotion
- YouTube
- Lifestyle
- Health
- Computer
- Téléphone
- Music
- Accounting
- Causes
- Networking
Lire la suite
How to Make Money from Facebook – A Step-by-Step Guide
Facebook offers numerous ways to earn money,...
What to do When working with the DOM
When working with the DOM (Document Object...
The Power of Video Marketing: How to Grow with YouTube & TikTok
In the digital era, video marketing has become...
The Role of Databases in Modern Applications
Databases are crucial in modern applications,...
Business Ideas As a Tech Professional
As a tech professional, you have a wide range...