How to Build a Responsive Navbar with HTML, CSS, & JavaScript
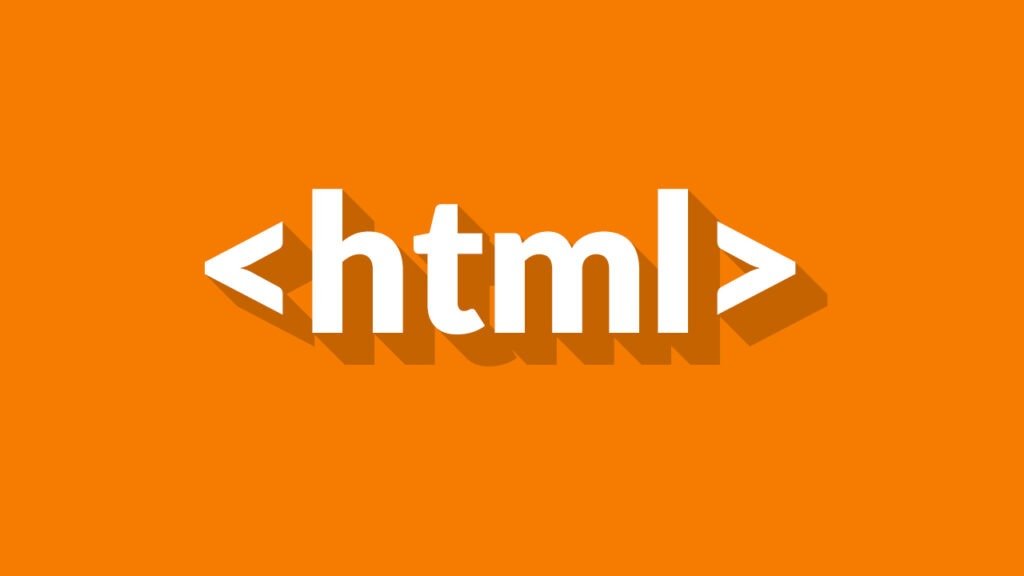
Here’s a detailed guide on How to Build a Responsive Navbar with HTML, CSS, & JavaScript, including explanations, keywords, and examples.
A Responsive Navigation Bar (Navbar) is a crucial component of modern web design. It allows users to navigate through different sections of a website smoothly on different screen sizes (desktop, tablet, and mobile).
Key Features of the Responsive Navbar
- Mobile-Friendly Design - Adjusts layout based on screen size.
- Hamburger Menu (Toggle Button) - Collapses into an icon on small screens.
- Dropdown Submenu - Allows for nested navigation.
- Sticky Navbar - Stays fixed at the top when scrolling.
- Dark Mode Toggle - Allows users to switch between light and dark themes.
- Smooth Animations - Enhances user experience.
- Search Bar Integration - Allows users to search within the site.
Step 1: HTML Structure
We start by writing a clean, semantic HTML structure.
Code: index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Navbar</title>
<link rel="stylesheet" href="styles.css">
<script defer src="script.js"></script>
</head>
<body>
<nav class="navbar">
<div class="logo">MySite</div>
<ul class="nav-links">
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li class="dropdown">
<a href="#">Services ▼</a>
<ul class="dropdown-menu">
<li><a href="#">Web Design</a></li>
<li><a href="#">SEO</a></li>
<li><a href="#">Marketing</a></li>
</ul>
</li>
<li><a href="#">Contact</a></li>
</ul>
<div class="hamburger">
☰
</div>
</nav>
</body>
</html>
Explanation:
<nav>
- The navigation bar wrapper.<div class="logo">
- Displays the brand name.<ul class="nav-links">
- Holds all navigation items.<li class="dropdown">
- Contains a submenu inside an unordered list (<ul class="dropdown-menu">
).<div class="hamburger">
- A toggle button for mobile screens.
Step 2: Styling with CSS
To make the navbar visually appealing and responsive, we use CSS.
Code: styles.css
/* General Styles */
body {
margin: 0;
font-family: Arial, sans-serif;
}
/* Navbar Styling */
.navbar {
display: flex;
justify-content: space-between;
align-items: center;
background: #333;
padding: 15px 20px;
color: white;
}
/* Logo */
.logo {
font-size: 1.5em;
font-weight: bold;
}
/* Navigation Links */
.nav-links {
list-style: none;
display: flex;
gap: 20px;
}
.nav-links li {
position: relative;
}
.nav-links a {
text-decoration: none;
color: white;
padding: 10px;
transition: 0.3s;
}
.nav-links a:hover {
background: #555;
border-radius: 5px;
}
/* Dropdown Menu */
.dropdown-menu {
display: none;
position: absolute;
background: #444;
list-style: none;
top: 35px;
left: 0;
padding: 10px;
border-radius: 5px;
}
.dropdown:hover .dropdown-menu {
display: block;
}
.dropdown-menu li {
padding: 10px;
}
.dropdown-menu li a {
color: white;
}
/* Hamburger Icon */
.hamburger {
font-size: 1.8em;
display: none;
cursor: pointer;
}
/* Responsive Design */
@media (max-width: 768px) {
.nav-links {
display: none;
flex-direction: column;
position: absolute;
top: 60px;
right: 0;
background: #333;
width: 100%;
text-align: center;
}
.nav-links.active {
display: flex;
}
.hamburger {
display: block;
}
}
Explanation:
- Flexbox (
display: flex;
) - Used for positioning elements efficiently. - Dropdown (
position: absolute;
) - The submenu appears when hovering. - Media Query (
@media (max-width: 768px)
) - Adjusts the navbar for mobile screens. - Hamburger Icon (
display: none;
) - Initially hidden but shown in mobile view.
Step 3: Adding JavaScript for Interactivity
We use JavaScript to toggle the mobile menu.
Code: script.js
document.addEventListener("DOMContentLoaded", () => {
const hamburger = document.querySelector(".hamburger");
const navLinks = document.querySelector(".nav-links");
hamburger.addEventListener("click", () => {
navLinks.classList.toggle("active");
});
});
Explanation:
document.querySelector()
- Selects the elements.addEventListener("click", function()")
- Triggers the toggle action when clicked.classList.toggle("active")
- Adds or removes the class.active
, which controls visibility.
Step 4: Enhancements
1. Sticky Navbar
Modify CSS to keep the navbar fixed when scrolling:
.navbar {
position: fixed;
width: 100%;
top: 0;
z-index: 1000;
}
body {
padding-top: 60px;
}
2. Dark Mode Toggle
Add a button to switch themes:
<button id="dark-mode-toggle">Dark Mode</button>
Modify JavaScript:
document.getElementById("dark-mode-toggle").addEventListener("click", () => {
document.body.classList.toggle("dark-mode");
});
Modify CSS:
.dark-mode {
background: #121212;
color: white;
}
Final Thoughts
By following this guide, you've built a fully functional responsive navbar that adapts to different devices, supports a dropdown menu, and includes a dark mode toggle. You can further enhance it by adding animations, a search bar, or even integrating it with a backend for dynamic content.
Key Takeaways
- HTML for structure
- CSS for styling & responsiveness
- JavaScript for interactivity
- Flexbox for layout
- Media Queries for responsiveness
- Artificial Intelligence (AI)
- Cybersecurity
- Blockchain & Cryptocurrencies
- Internet of Things
- Cloud Computing
- Big Data & Analytics
- Virtual Reality
- 5G & Future Connectivity
- Robotics & Automation
- Software Development & Programming
- Tech Hardware & Devices
- Tech in Healthcare
- Tech in Business
- Gaming Technologies
- Tech in Education
- Machine Learning (ML)
- Blogging
- Affiliate Marketing
- Make Money
- Digital Marketing
- Product Review
- Social Media
- Excel
- Graphics design
- Freelancing/Consulting
- FinTech (Financial Technology)
- E-commerce and Digital Marketing
- Business
- Sport
- Self Development
- Tips to Success
- Video Editing
- Photo Editing
- Website Promotion
- YouTube
- Lifestyle
- Health
- Computer
- Téléphone
- Music
- Accounting
- Causes
- Networking