HTML
How to Create a Responsive Navigation Bar (navbar) Using HTML, CSS, and JavaScript
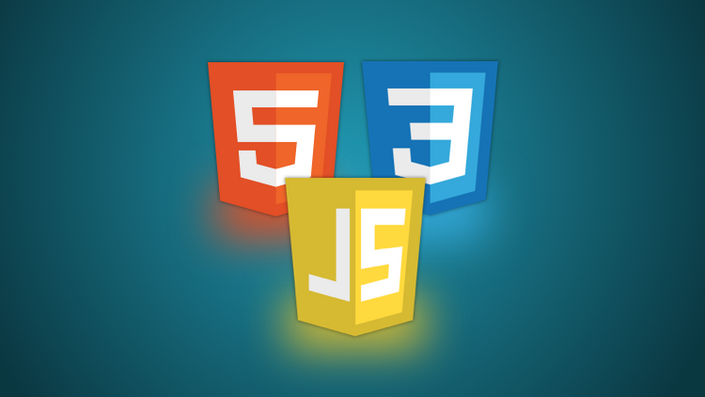
Creating a responsive navigation bar (navbar) using HTML, CSS, and JavaScript ensures that your website's menu adjusts seamlessly across different screen sizes, from desktops to mobile devices. Below is a step-by-step guide to building a responsive navbar, including key points, explanations, and full source code.
Key Points of a Responsive Navbar
- Structure with HTML: Use the
<nav>
element with a list (<ul>
) for menu items. - Styling with CSS: Implement flexbox for layout, media queries for responsiveness, and animations for a smooth experience.
- Interactivity with JavaScript: Use JavaScript to toggle the mobile menu when clicking a button (hamburger icon).
- Mobile-Friendly Design: Hide the menu initially on small screens and show it when the button is clicked.
- Accessibility Considerations: Use
aria-labels
for screen readers.
Step 1: HTML Structure
We will create a simple navigation bar with a logo, menu items, and a hamburger icon for mobile devices.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Navbar</title>
<link rel="stylesheet" href="styles.css">
<script defer src="script.js"></script>
</head>
<body>
<nav class="navbar">
<div class="logo">MyLogo</div>
<ul class="nav-links">
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Services</a></li>
<li><a href="#">Contact</a></li>
</ul>
<div class="hamburger">
<span></span>
<span></span>
<span></span>
</div>
</nav>
</body>
</html>
Explanation
- The
<nav>
element wraps the logo, navigation links, and hamburger icon. - The
<ul class="nav-links">
contains the menu items. - The
<div class="hamburger">
is used for the hamburger icon, which will toggle the menu on small screens.
Step 2: CSS Styling
Now, let’s style the navbar for both desktop and mobile views.
/* styles.css */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: Arial, sans-serif;
}
/* Navbar styling */
.navbar {
display: flex;
justify-content: space-between;
align-items: center;
background-color: #333;
padding: 15px 20px;
color: white;
}
.logo {
font-size: 24px;
font-weight: bold;
}
.nav-links {
list-style: none;
display: flex;
}
.nav-links li {
margin: 0 15px;
}
.nav-links a {
text-decoration: none;
color: white;
font-size: 18px;
transition: color 0.3s ease;
}
.nav-links a:hover {
color: #f4a261;
}
/* Hamburger Menu */
.hamburger {
display: none;
flex-direction: column;
cursor: pointer;
}
.hamburger span {
width: 30px;
height: 3px;
background: white;
margin: 5px 0;
transition: transform 0.3s ease;
}
/* Responsive Design */
@media (max-width: 768px) {
.nav-links {
display: none;
flex-direction: column;
position: absolute;
top: 60px;
left: 0;
width: 100%;
background: #333;
padding: 10px 0;
text-align: center;
}
.nav-links.active {
display: flex;
}
.nav-links li {
margin: 10px 0;
}
.hamburger {
display: flex;
}
/* Rotate Hamburger Icon */
.hamburger.active span:nth-child(1) {
transform: translateY(8px) rotate(45deg);
}
.hamburger.active span:nth-child(2) {
opacity: 0;
}
.hamburger.active span:nth-child(3) {
transform: translateY(-8px) rotate(-45deg);
}
}
Explanation
- The
.navbar
is a flexbox container to align the logo and menu items. .hamburger
is hidden by default and displayed only on small screens (@media (max-width: 768px)
)..nav-links.active
is used to show the menu when clicked.- Animations are added to smoothly transition the menu and hamburger icon.
Step 3: JavaScript for Interactivity
Finally, we use JavaScript to toggle the menu visibility when the hamburger icon is clicked.
// script.js
document.addEventListener("DOMContentLoaded", function () {
const hamburger = document.querySelector(".hamburger");
const navLinks = document.querySelector(".nav-links");
hamburger.addEventListener("click", () => {
navLinks.classList.toggle("active");
hamburger.classList.toggle("active");
});
});
Explanation
- Selects the hamburger icon and nav-links.
- Adds a
click
event to toggle theactive
class, which:- Shows or hides the navigation menu.
- Animates the hamburger icon into a close (
X
) shape.
Final Output
✅ The navbar is now fully responsive:
- Desktop View: Menu appears normally.
- Mobile View:
- The menu is hidden.
- Clicking the hamburger icon shows the menu.
- The hamburger transforms into an 'X' when active.
Enhancements
💡 You can improve the navbar further by:
- Adding dropdown menus for multi-level navigation.
- Enhancing animations with smooth transitions.
- Using JavaScript local storage to remember the menu state.
Would you like any additional features, such as submenus, animations, or a dark mode toggle?