Setting Up a Node.js Environment
Posté 2025-02-23 21:10:37
0
340
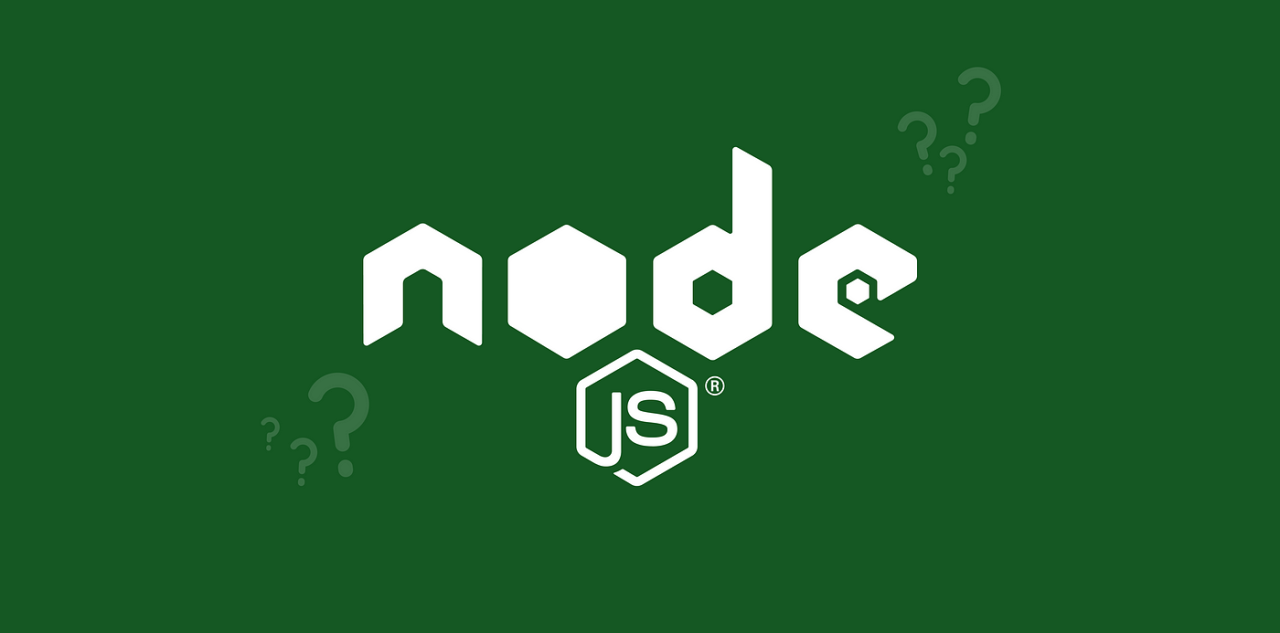
Setting up Node.js involves installing Node.js itself, configuring your development environment, and ensuring you have the necessary tools to start coding.
Step 1: Download and Install Node.js
- Go to the official Node.js website → https://nodejs.org
- Choose the right version:
- LTS (Long-Term Support) – Recommended for most users, especially for production.
- Current – Latest features but might be unstable.
- Download and install the appropriate version for your OS (Windows, macOS, Linux).
- Verify installation by running the following commands in your terminal or command prompt:
node -v # Check Node.js version npm -v # Check npm (Node Package Manager) version
Step 2: Install a Code Editor (Optional, but Recommended)
A good code editor makes development easier. Some popular choices:
- VS Code (Recommended) – Download
- Sublime Text
- Atom
If using VS Code, install the "Node.js Extension Pack" for a better experience.
Step 3: Initialize a Node.js Project
- Create a new project folder:
mkdir my-node-app cd my-node-app
- Initialize a Node.js project:
This creates anpm init -y
package.json
file that stores project information and dependencies.
Step 4: Install Dependencies
Install packages using npm
(Node Package Manager). Example:
npm install express # Installs Express.js for building web applications
List installed packages:
npm list
Step 5: Create and Run a Simple Node.js Script
- Create a file
app.js
and add:console.log("Hello, Node.js!");
- Run the script:
node app.js
Step 6: Set Up a Basic Web Server (Optional)
Create a simple HTTP server using Node.js:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, Node.js Server!');
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000');
});
Run the server:
node app.js
Open your browser and visit http://localhost:3000.
Step 7: Use Nodemon for Auto-Restart (Optional)
Instead of restarting the server manually after every change, install nodemon:
npm install -g nodemon
Run your app with:
nodemon app.js
Step 8: Explore More!
- Learn about Express.js for web apps.
- Use MongoDB or MySQL for databases.
- Try REST APIs and real-time applications.
Rechercher
Sellect from all Catégories bellow ⇓
- Artificial Intelligence (AI)
- Cybersecurity
- Blockchain & Cryptocurrencies
- Internet of Things
- Cloud Computing
- Big Data & Analytics
- Virtual Reality
- 5G & Future Connectivity
- Robotics & Automation
- Software Development & Programming
- Tech Hardware & Devices
- Tech in Healthcare
- Tech in Business
- Gaming Technologies
- Tech in Education
- Machine Learning (ML)
- Blogging
- Affiliate Marketing
- Make Money
- Digital Marketing
- Product Review
- Social Media
- Excel
- Graphics design
- Freelancing/Consulting
- FinTech (Financial Technology)
- E-commerce and Digital Marketing
- Business
- Sport
- Self Development
- Tips to Success
- Video Editing
- Photo Editing
- Website Promotion
- YouTube
- Lifestyle
- Health
- Computer
- Téléphone
- Music
- Accounting
- Causes
- Networking
Lire la suite
Top 10 Ethical Hacking Terms You Must Know
Here are the Top 10 Ethical Hacking Terms You...
Introduction to Computer Science
Computer Science is a vast and dynamic field...
Deep Dive Comparison Between React Native and Flutter
Here's a deep dive comparison between React...
How to Determine Which Tech Area to Focus on as a Beginner
Choosing the right tech area to focus on as a...
PWA vs Native App: Which One to Build?
Choosing between a Progressive Web App (PWA)...